6 Animations Katas
6.1 Animations 1 Kata (difficulty = 3)
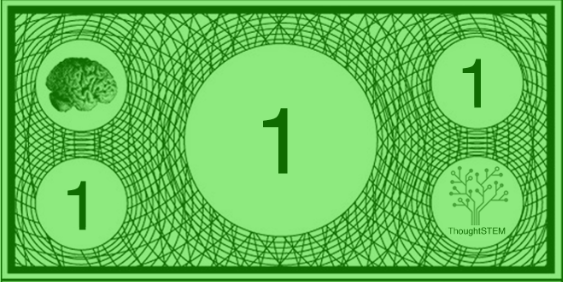
read
Code a scene with a random-colored cylinder that continuously grows and shrinks.
code
#lang 3d-exploration (define (my-cylinder) (basic-cylinder #:color (random-color) #:animations-list (do-many (animate-scale #:to 5)))) (exploration-scene #:ground-objects (list (my-cylinder)))
6.2 Animations 2 Kata (difficulty = 3)
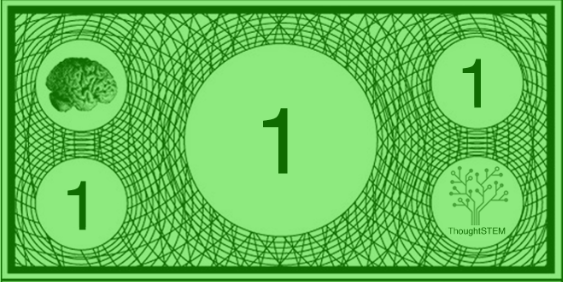
read
Code a scene with a cone that moves back and forth several times.
code
#lang 3d-exploration (define (my-cone) (basic-cone #:animations-list (do-many (animate-position #:loops 5 #:to (position 0 0 0))))) (exploration-scene #:sky-objects (list (my-cone)))
6.3 Animations 3 Kata (difficulty = 3)
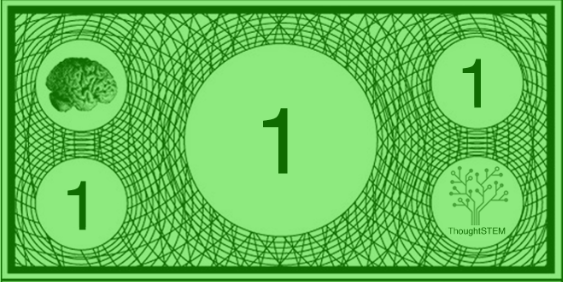
Tip: basic-box also has these keywords: depth, height, and width.
read
Code a scene with a random-colored box that grows and shrinks while rotating back and forth.
code
#lang 3d-exploration (define (my-box) (basic-box #:color (random-color) #:animations-list (do-many (animate-rotation) (animate-scale #:to 3)))) (exploration-scene #:ground-objects (list (my-box)))
6.4 Animations 4 Kata (difficulty = 4)
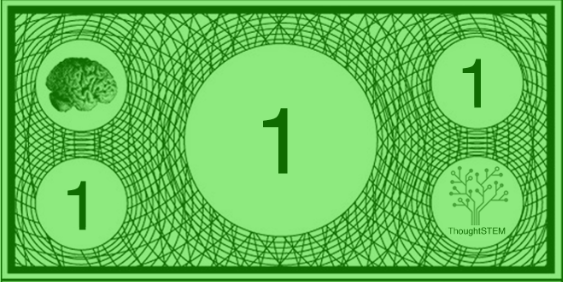
read
Code a scene with an Earth sphere rotating around a Sun sphere.
code
#lang 3d-exploration (define (earth) (basic-sphere #:position (position 25 0 0) #:texture earth-tex)) (define (sun) (basic-sphere #:texture sun-tex #:radius 5 #:animations-list (do-many (animate-rotation)) #:components-list (list (earth)))) (exploration-scene #:sky-objects (list (sun)))
6.5 Animations 5 Kata (difficulty = 3)
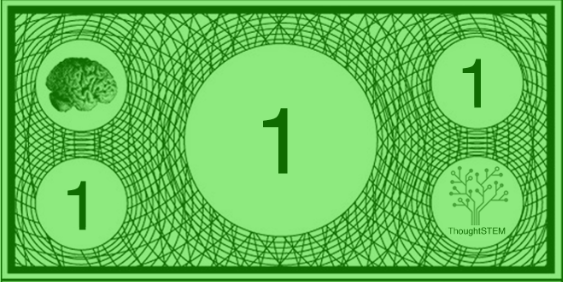
read
Code a scene with a bird flying away.
code
#lang 3d-exploration (define (my-model) (3d-model #:model bird #:position (position 0 0 0) #:animations-list (do-many (animate-position #:to (position 100 100 100) #:loops 0)))) (exploration-scene #:ground-objects (list (my-model)))