3 Ground Objects Katas
3.1 Ground Objects 1 Kata (difficulty = 2)
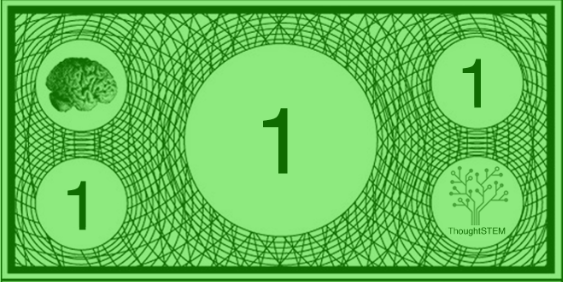
Tip: Try these other 3D objects: basic-box, basic-cone, basic-cylinder, basic-dodecahedron, basic-icosahedron, basic-octahedron, or basic-tetrahedron.
read
Code a scene with a red, semi-transparent sphere on the ground.
code
#lang 3d-exploration (define (my-sphere) (basic-sphere #:color 'red #:opacity 0.75)) (exploration-scene #:ground-objects (list (my-sphere)))
3.2 Ground Objects 2 Kata (difficulty = 3)
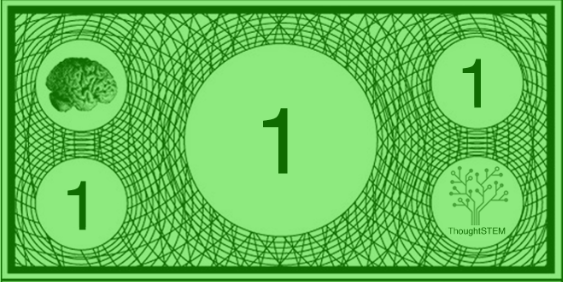
Tip: All the 3D objects share these keywords: position, rotation, scale, color, opacity, texture, on-mouse-enter, on-mouse-leave, on-mouse-click, animations-list, and components-list.
read
Code a scene with a large cylinder and a large blue box.
code
#lang 3d-exploration (define (my-cylinder) (basic-cylinder #:radius 3 #:height 5)) (define (my-box) (basic-box #:scale 5 #:color 'blue)) (exploration-scene #:ground-objects (list (my-cylinder) (my-box)))
3.3 Ground Objects 3 Kata (difficulty = 2)
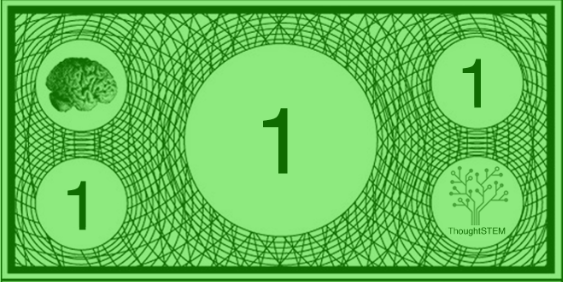
Tip: Try passing (random-color) to the color keyword.
read
Code a scene with 3 large orange oceans.
code
#lang 3d-exploration (define (my-ocean) (basic-ocean #:color 'orange #:width 30 #:depth 20)) (exploration-scene #:ground-objects (list (my-ocean) (my-ocean) (my-ocean)))
3.4 Ground Objects 4 Kata (difficulty = 2)
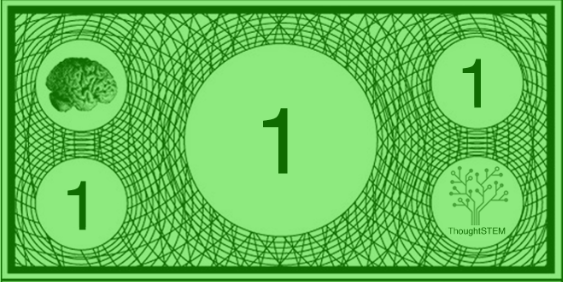
Tip: basic-sphere also has the #:radius keyword as well as these other 3D objects basic-cylinder, basic-dodecahedron, basic-icosahedron, basic-octahedron, basic-sphere, and basic-tetrahedron.
read
Code a scene with a sphere that changes color when you click on it.
code
#lang 3d-exploration (define (my-sphere) (basic-sphere #:color 'red #:on-mouse-click (do-many (color 'blue)))) (exploration-scene #:ground-objects (list (my-sphere)))
3.5 Ground Objects 5 Kata (difficulty = 4)
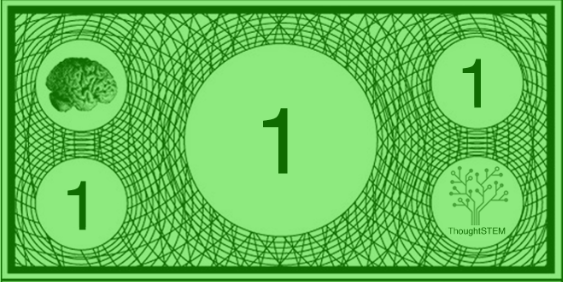
Tip: basic-cylinder also has the #:height keyword.
read
Code a scene with a cylinder that changes color and grows when look at and reverts back when not looked at.
code
#lang 3d-exploration (define (my-cylinder) (basic-cylinder #:color 'orange #:on-mouse-enter (do-many (color 'yellow) (radius 5)) #:on-mouse-leave (do-many (color 'orange) (radius 1)))) (exploration-scene #:ground-objects (list (my-cylinder)))