3 Enemy Katas
3.1 Enemy Kata (difficulty = 1)
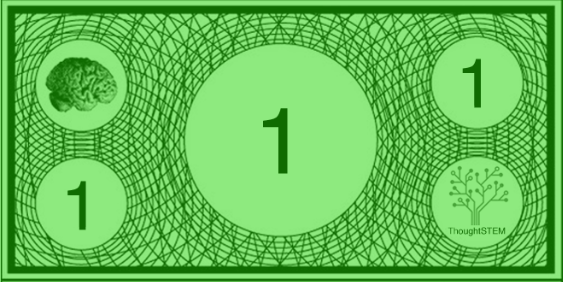
read
Code a game with an enemy.
code
#lang battlearena (battlearena-game #:enemy-list (list (basic-enemy)))
3.2 Enemy Kata (difficulty = 1)
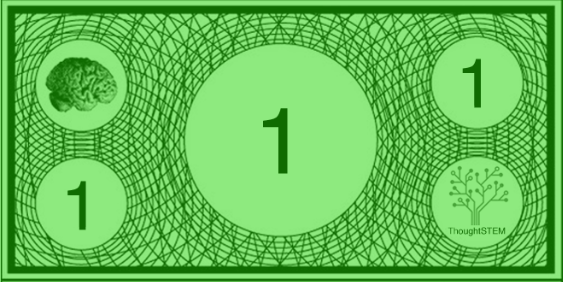
Tip: Without the curry, the game will have 10 of the same enemy.
With curry, the game will have 10 different enemies.
read
Code a game with 10 random enemies
code
#lang battlearena (battlearena-game #:enemy-list (list (curry basic-enemy #:amount-in-world 10)))
3.3 Enemy Kata (difficulty = 2)
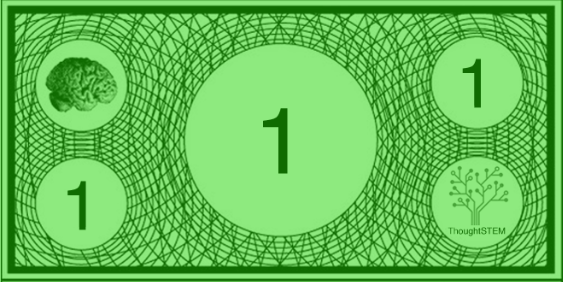
Tip: The options for #:ai are ’easy, ’medium or ’hard.
read
Code a game with several enemies that have moderate intelligence.
code
#lang battlearena (define (my-enemy) (basic-enemy #:sprite darkknight-sprite #:ai 'medium #:amount-in-world 5)) (battlearena-game #:enemy-list (list (my-enemy)))
3.4 Enemy Kata (difficulty = 3)
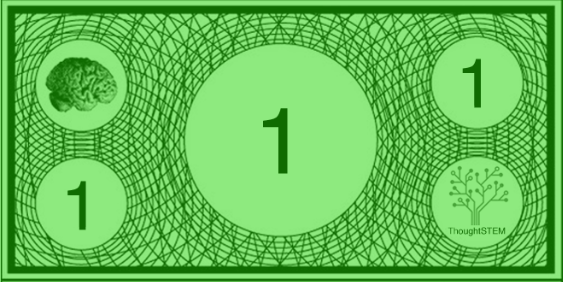
read
Code a game with 8 enemies: 5 weak with low intelligence, and 3 strong with moderate intelligence. Choose your own sprites.
code
#lang battlearena (define (easy-enemy) (basic-enemy #:ai 'easy #:sprite wizard-sprite #:health 50 #:amount-in-world 5)) (define (medium-enemy) (basic-enemy #:ai 'medium #:sprite darkknight-sprite #:health 200 #:amount-in-world 3)) (battlearena-game #:enemy-list (list (easy-enemy) (medium-enemy)))
3.5 Enemy Kata (difficulty = 2)
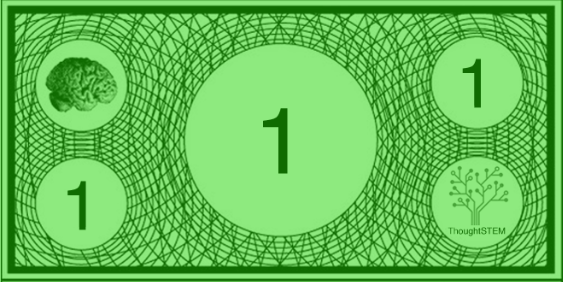
read
Code a game with 5 high intelligence enemies that have a weapon that does 50 damage.
code
#lang battlearena (define (hard-enemy) (basic-enemy #:ai 'hard #:sprite pirategirl-sprite #:amount-in-world 5 #:weapon (repeater #:damage 50))) (battlearena-game #:avatar (basic-avatar) #:enemy-list (list (hard-enemy)))