5 Powerup Katas
5.1 Health Kata (difficulty = 1)
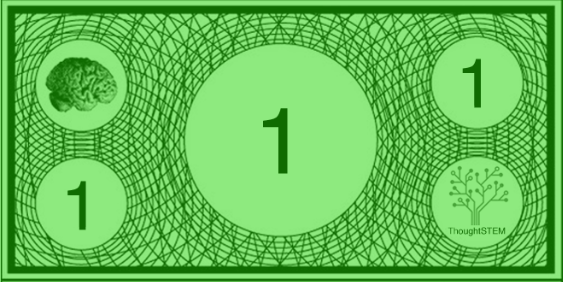
Tip: You can also try set-health-to.
read
Code a game with a "Health Power-up" item that increases your health. Customize the icon and make it respawnable.
code
#lang battlearena (battlearena-game #:item-list (list (basic-item #:name "Health Power-up" #:icon (make-icon "HP" 'green 'white) #:on-use (change-health-by 50) #:respawn? #t)))
5.2 Shield Kata (difficulty = 1)
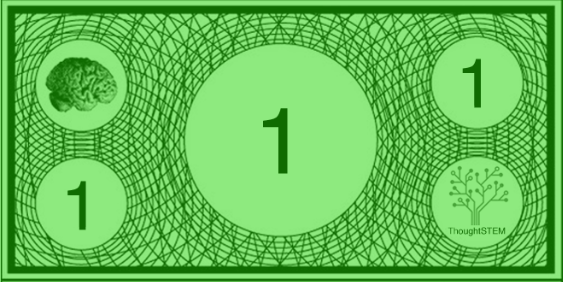
Tip: You can also try change-shield-by.
read
Code a game with a "Max Shield Power-up" item that sets your shield to 100. Customize the icon.
code
#lang battlearena (battlearena-game #:item-list (list (basic-item #:name "Max Shield Power-up" #:icon (make-icon "MSP" 'blue 'white) #:on-use (set-shield-to 100))))
5.3 Boost Kata (difficulty = 2)
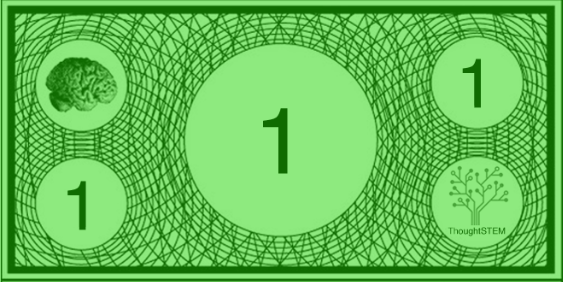
Tip: You can also try set-damage-to and multiply-damage-by.
read
Code a game with a "Damage Boost" item that temporarily increases the damage of your weapon. Customize the icon.
code
#lang battlearena (battlearena-game #:weapon-list (list (repeater)) #:item-list (list (basic-item #:name "Damage Boost" #:icon (make-icon "DB" 'orangered) #:on-use (change-damage-by 1000 #:for 200))))
5.4 Boost Kata (difficulty = 1)
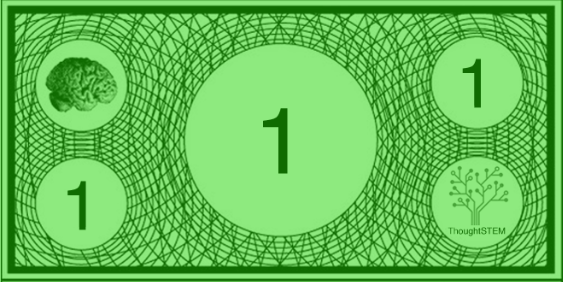
Tip: You can also try set-speed-to and change-speed-by. The default movement speed is 10.
read
Code a game with a temporary "Speed Boost" item with a custom icon.
code
#lang battlearena (battlearena-game #:item-list (list (basic-item #:name "Speed Boost" #:icon (make-icon "SB" 'yellow) #:on-use (multiply-speed-by 2 #:for 200))))
5.5 Size Kata (difficulty = 1)
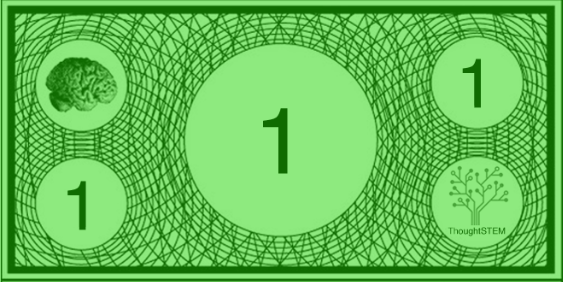
read
Code a game with a "Grow Power-up" item that makes you bigger. Customize the icon.
code
#lang battlearena (battlearena-game #:item-list (list (basic-item #:name "Grow Power-up" #:icon (make-icon "BIG" 'red 'white) #:on-use (scale-sprite 2 #:for 100))))
5.6 Force Field Kata (difficulty = 2)
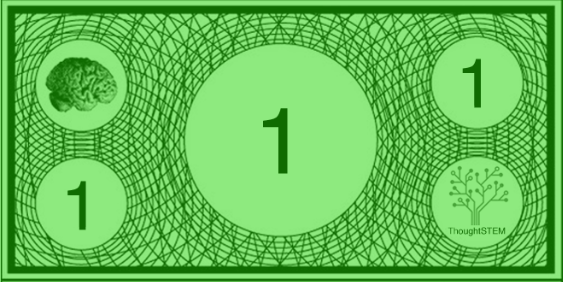
Tip: The default duration is 500.
read
Code a game with a "Force Field" item that lasts for a long time. Customize the icon.
code
#lang battlearena (battlearena-game #:item-list (list (basic-item #:name "Force Field" #:icon (make-icon "FF") #:on-use (spawn (force-field #:duration 1000)))))
5.7 Force Field Kata (difficulty = 3)
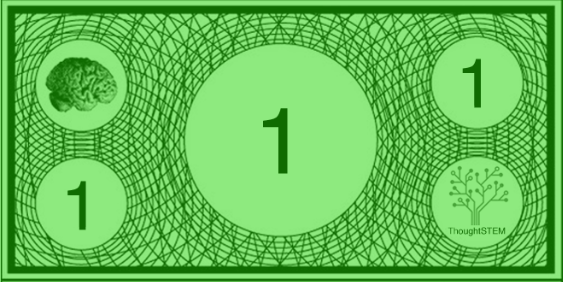
Tip: Setting #:allow-friendly-dart? to #t will allow you to shoot through your force-field.
read
Code a game with a "Force Field" item that has a custom icon. Customize the duration and allow the player to shoot out of it.
code
#lang battlearena (define (force-field-item) (basic-item #:name "Force Field" #:icon (make-icon "FF") #:on-use (spawn (force-field #:allow-friendly-dart? #t #:duration 3000)))) (battlearena-game #:item-list (list (force-field-item)))