4 Weapon Katas
4.1 Sword Kata (difficulty = 1)
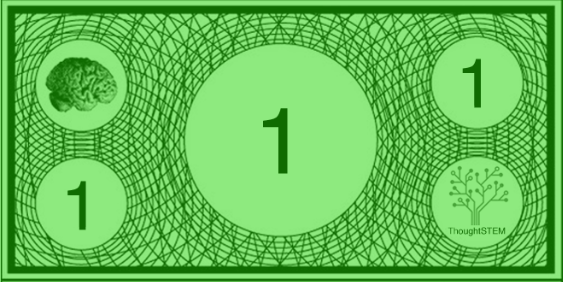
Tip: The default damage is 25
read
Code a game with a sword that does 50 damage.
code
#lang battlearena (battlearena-game #:weapon-list (list (sword #:name "Heavy Sword" #:damage 50)))
4.2 Spear Kata (difficulty = 2)
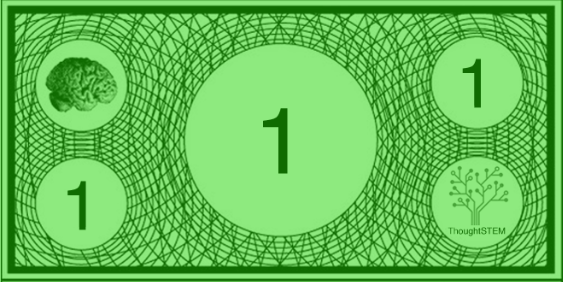
Rarity Rules:
’common = 5 total
’uncommon = 4 total
’rare = 3 total
’epic = 2 total
’legendary = 1 total
read
Code a game with an epic rarity spear that does 50 damage.
code
#lang battlearena (define (my-spear) (spear #:name "Heavy Spear" #:damage 50 #:rarity 'epic)) (battlearena-game #:weapon-list (list (my-spear)))
4.3 Fire Magic Kata (difficulty = 2)
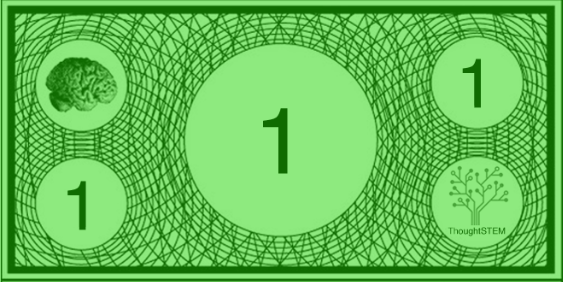
Tip: You can also try ice-magic, sword-magic, ring-of-fire, ring-of-ice, or ring-of-blades.
read
Code a game with fire magic that does 20 damage.
code
#lang battlearena (define (my-weapon) (fire-magic #:name "Heavy Fire Magic" #:damage 20)) (battlearena-game #:weapon-list (list (my-weapon)))
4.4 Fire Magic Kata (difficulty = 2)
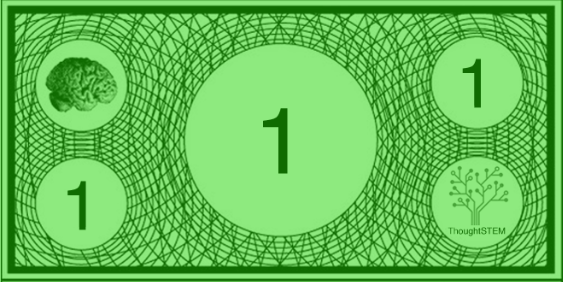
Tip: If you use ring-of-fire, ring-of-ice, or ring-of-blades, you must change #:range to #:duration.
read
Code a game with a legendary fire magic with a customized sprite, damage, speed, and range.
code
#lang battlearena (define (my-weapon) (fire-magic #:name "Fire Magic" #:icon (make-icon "FM" 'red) #:color 'green #:damage 20 #:speed 10 #:range 50 #:rarity 'legendary)) (battlearena-game #:weapon-list (list (my-weapon)))
4.5 Single Shot Kata (difficulty = 1)
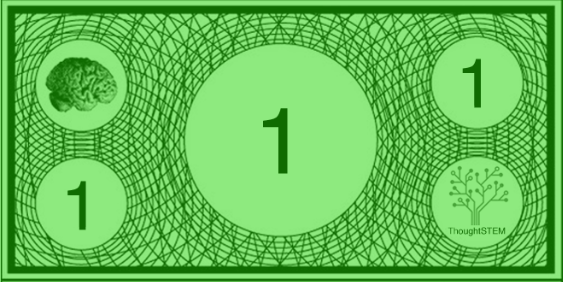
Tip: #:rapid-fire? defaults to #t. True (#t) means that you can hold the fire button to shoot.
read
Code a game with a weapon that fires only once per click.
code
#lang battlearena (battlearena-game #:weapon-list (list (repeater #:name "Single Shot" #:icon (make-icon "SS") #:rapid-fire? #f)))
4.6 Spread Shot Kata (difficulty = 2)
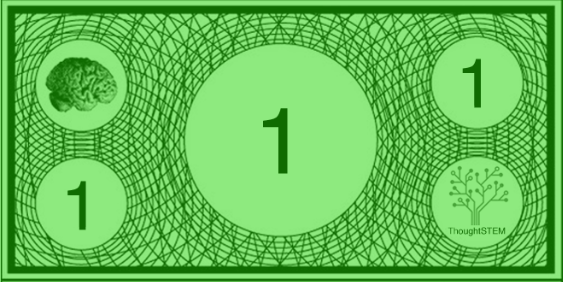
Tip: Default damage, durability, and speed are 10, 10, and 10. A durabilty of 20 means each dart can hit 2 targets.
read
Code a game with a powerful, fast repeater that shoots multiple darts. Customize the name and the icon.
code
#lang battlearena (define (my-weapon) (repeater #:name "Spread Shot" #:icon (make-icon "SPR") #:fire-mode 'spread #:damage 20 #:speed 15)) (battlearena-game #:weapon-list (list (my-weapon)))
4.7 Homing Repeater Kata (difficulty = 2)
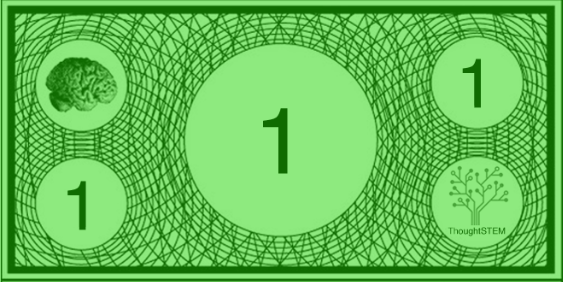
Rarity Rules:
’common = 5 total
’uncommon = 4 total
’rare = 3 total
’epic = 2 total
’legendary = 1 total
read
Code a game with a rare weapon that fires homing darts with customized sprite, damage, speed, and range.
code
#lang battlearena (define (homing-repeater) (repeater #:name "Homing Repeater" #:icon (make-icon "HR") #:fire-mode 'homing #:rarity 'rare #:sprite (rectangle 10 2 'solid 'pink) #:damage 50 #:speed 8 #:range 40)) (battlearena-game #:weapon-list (list (homing-repeater)))