4 Enemy Katas
4.1 Enemy Kata (difficulty = 1)
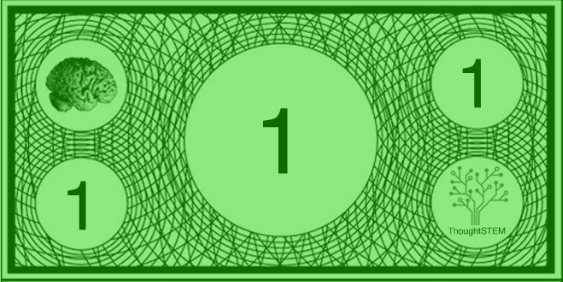
read
Code a game with an enemy.
code
#lang survival (survival-game #:enemy-list (list (basic-enemy)))
4.2 Enemy Kata (difficulty = 1)
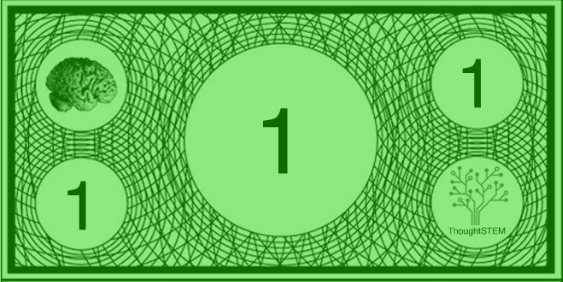
Tip: Without the curry, the game will have 10 of the same enemy. With curry, the game will have 10 different enemies.
read
Code a game with 10 random enemies.
code
#lang survival (survival-game #:enemy-list (list (basic-enemy #:amount-in-world 10)))
4.3 Enemy Kata (difficulty = 2)
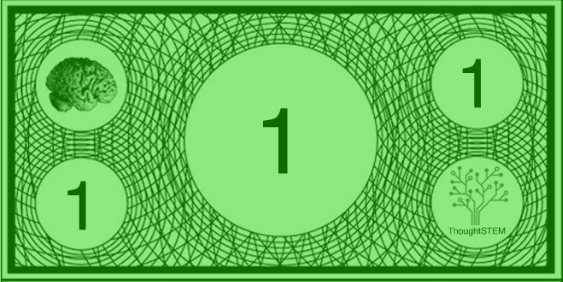
Tip: The options for #:ai are ’easy, ’medium and ’hard.
read
Code a game with 5 bat enemies with medium AI.
code
#lang survival (define (my-enemy) (basic-enemy #:ai 'medium #:sprite bat-sprite #:amount-in-world 5)) (survival-game #:enemy-list (list (my-enemy)))
4.4 Enemy Kata (difficulty = 3)
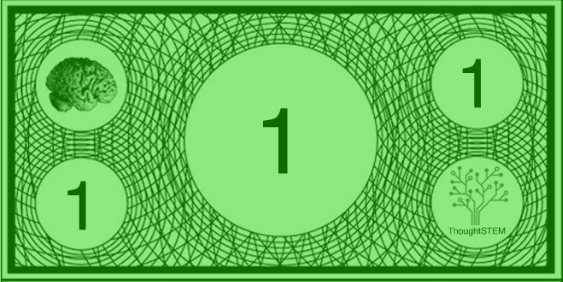
read
Code a game with 10 total mobs: half are not-smart slime, and half are smarter bats who only appear at night.
code
#lang survival (define (easy-enemy) (basic-enemy #:ai 'easy #:sprite slime-sprite #:amount-in-world 5)) (define (medium-enemy) (basic-enemy #:ai 'medium #:sprite bat-sprite #:amount-in-world 5 #:night-only? #t)) (survival-game #:enemy-list (list (easy-enemy) (medium-enemy)))
4.5 Enemy Kata (difficulty = 2)
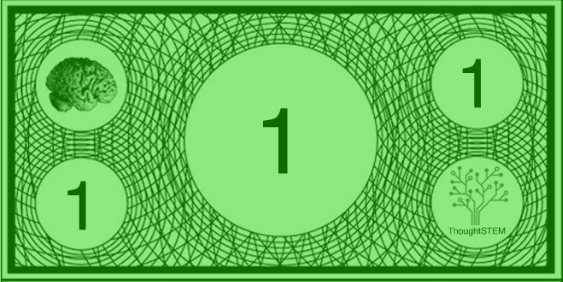
read
Code a game with 5 hard bat enemies that spit acid with 50 damage.
code
#lang survival (define (hard-enemy) (basic-enemy #:ai 'hard #:sprite snake-sprite #:amount-in-world 5 #:weapon (acid-spitter #:damage 50))) (survival-game #:enemy-list (list (hard-enemy)))
4.6 Enemy Kata (difficulty = 5)
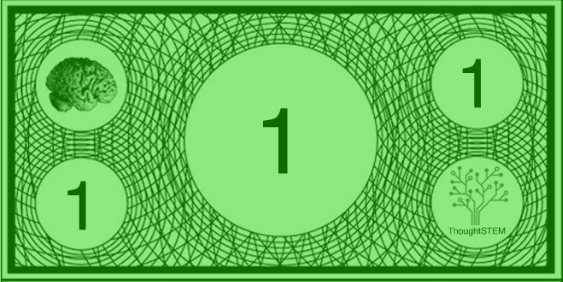
read
Code a game with 5 slimes with ’easy ai, 3 snakes with ’medium ai, and 1 bat with ’hard ai that only shows up at night and does 50 damage.
code
#lang survival (define (easy-enemy) (basic-enemy #:ai 'easy #:sprite slime-sprite #:amount-in-world 5)) (define (medium-enemy) (basic-enemy #:ai 'medium #:sprite snake-sprite #:amount-in-world 3)) (define (hard-enemy) (basic-enemy #:ai 'hard #:sprite bat-sprite #:amount-in-world 1 #:night-only? #t #:weapon (acid-spitter #:damage 50))) (survival-game #:enemy-list (list (easy-enemy) (medium-enemy) (hard-enemy)))