3 Food Katas
3.1 Food Kata (difficulty = 1)
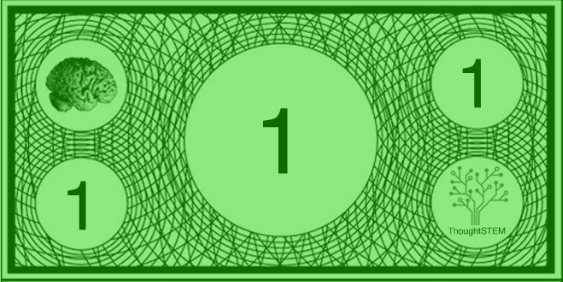
Tip: By default, there will be only 1 custom-food in world.
read
Code a game with 10 food items in the world.
code
#lang survival (survival-game #:food-list (list (basic-food #:amount-in-world 10)))
3.2 Food Kata (difficulty = 2)
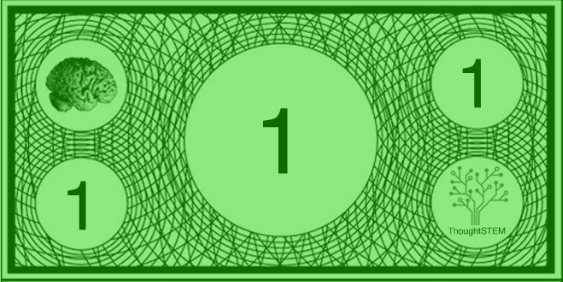
Tip: By default, custom-food will heal by 10.
read
Code a game with exactly 2 custom foods that heal you by 20.
code
#lang survival (define (my-food) (basic-food #:amount-in-world 2 #:heals-by 20)) (survival-game #:food-list (list (my-food)))
3.3 Food Kata (difficulty = 2)
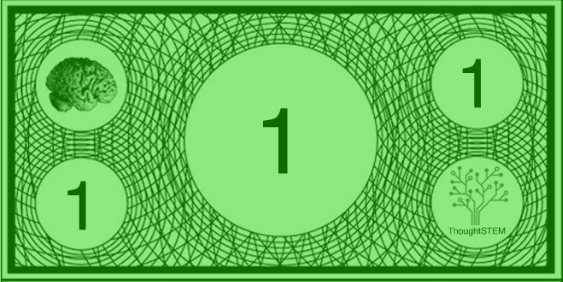
read
Code a game with a couple of apples with extra healing power.
code
#lang survival (define (my-food) (basic-food #:sprite apples-sprite #:name "Apples" #:amount-in-world 2 #:heals-by 20)) (survival-game #:food-list (list (my-food)))
3.4 Food Kata (difficulty = 3)
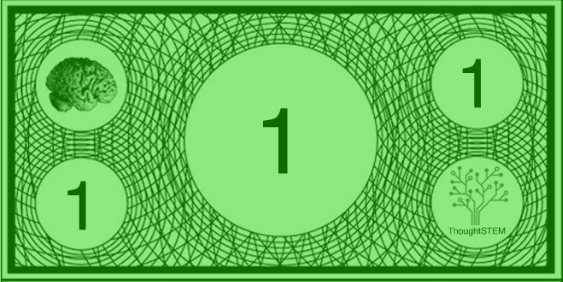
read
Code a game with 2 different food types: common apples with low-healing power and one very rare cherry with high-healing power.
code
#lang survival (define (my-food) (basic-food #:sprite apples-sprite #:name "Apples" #:amount-in-world 15 #:heals-by 5)) (define (special-food) (basic-food #:sprite cherry-sprite #:name "Cherry" #:amount-in-world 1 #:heals-by 50 #:respawn? #f)) (survival-game #:food-list (list (my-food) (special-food)))
3.5 Food Kata (difficulty = 2)
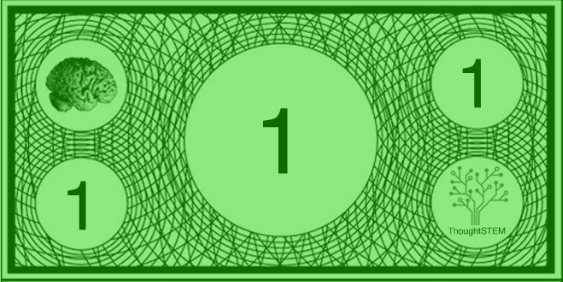
Tip: The default starvation rate is 50.
read
Code a game with a very fast starvation rate and lots of cherries with high-healing power.
code
#lang survival (define (my-food) (basic-food #:sprite cherry-sprite #:name "Cherries" #:amount-in-world 20 #:heals-by 50)) (survival-game #:food-list (list (my-food)) #:starvation-rate 100)
3.6 Food Kata (difficulty = 4)
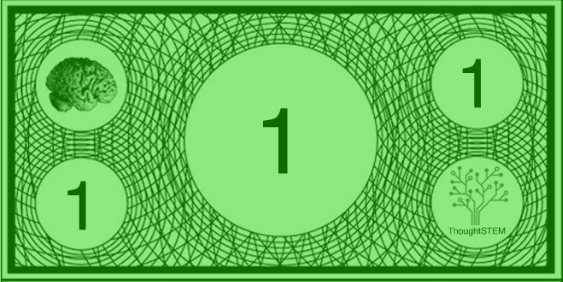
read
Code a game with 3 food types: common cherries, trick smores that deplete your health, and a single high-healing carrot that spawns, only once, on a specific tile.
code
#lang survival (define (basic-cherry) (basic-food #:sprite cherry-sprite #:name "Cherries" #:amount-in-world 15)) (define (basic-smores) (basic-food #:sprite smores-sprite #:name "Smores" #:heals-by -10)) (define (special-carrot) (basic-food #:sprite carrot-sprite #:name "Carrots" #:tile 4 #:amount-in-world 1 #:heals-by 50 #:respawn? #f)) (survival-game #:food-list (list (basic-cherry) (basic-smores) (special-carrot)))