7 Extra Katas
7.1 Coin Kata (difficulty = 1)
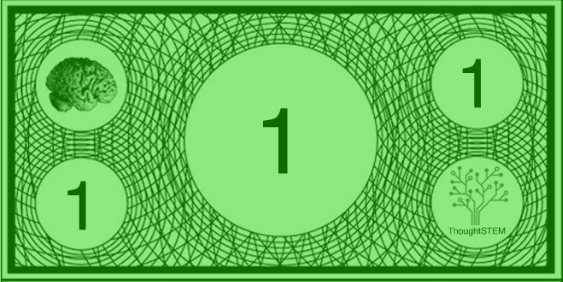
read
Code a game with collectible coins.
code
#lang survival (survival-game #:coin-list (list (basic-coin)))
7.2 Coin Kata (difficulty = 2)
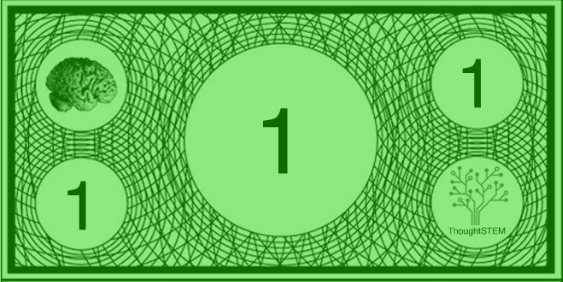
Tip: The default value of custom-coin is 10.
read
Code a game with very valuable collectible coins.
code
#lang survival (define (my-coin) (basic-coin #:value 500)) (survival-game #:coin-list (list (my-coin)))
7.3 Coin Kata (difficulty = 2)
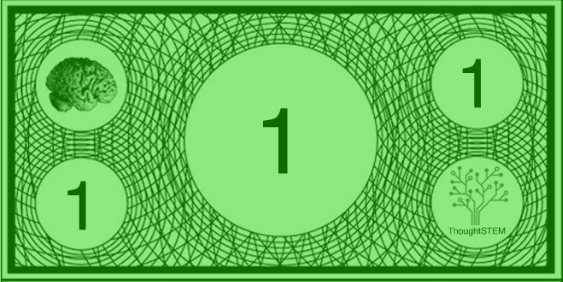
read
Code a game with many valuable silver coins.
code
#lang survival (define (my-coin) (basic-coin #:sprite silvercoin-sprite #:name "Silver Coin" #:value 100 #:amount-in-world 20)) (survival-game #:coin-list (list (my-coin)))
7.4 Coin Kata (difficulty = 3)
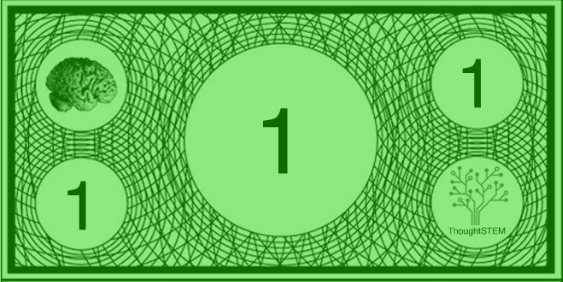
read
Code a game with a silver coin, and a single, extremely valuable gold coin that only appears once.
code
#lang survival (define (my-coin) (basic-coin #:sprite silvercoin-sprite #:name "Silver Coin")) (define (my-special-coin) (basic-coin #:sprite goldcoin-sprite #:name "Gold Coin" #:value 1000 #:amount-in-world 1 #:respawn? #f)) (survival-game #:coin-list (list (my-coin) (my-special-coin)))
7.5 Coin Kata (difficulty = 3)
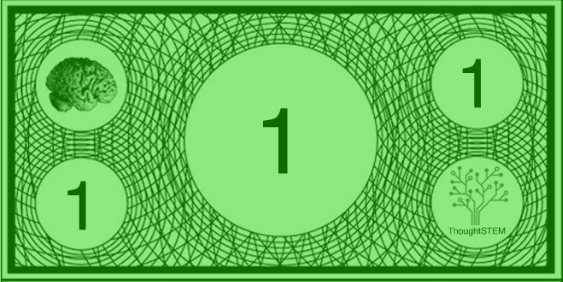
read
Code a game with 3 collectible coins: a basic coin; a rarer, more valuable silver coin; and an extremely rare and valuable gold coin.
code
#lang survival (define (silver-coin) (basic-coin #:sprite silvercoin-sprite #:name "Silver Coin" #:value 500 #:amount-in-world 5)) (define (gold-coin) (basic-coin #:sprite goldcoin-sprite #:name "Gold Coin" #:value 1000 #:amount-in-world 1 #:respawn? #f)) (survival-game #:coin-list (list (basic-coin) (silver-coin) (gold-coin)))
7.6 Weapon Crafter Kata (difficulty = 2)
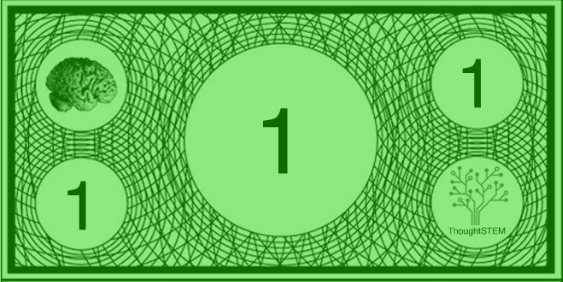
read
Code a game with a crafter that builds swords.
code
#lang survival (define my-sword-recipe (recipe #:product (sword) #:build-time 20)) (survival-game #:crafter-list (list (basic-crafter #:sprite woodtable-sprite #:recipe-list (list my-sword-recipe))))
7.7 Weapon Crafter Kata (difficulty = 2)
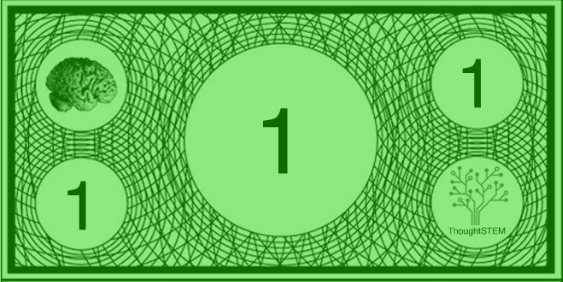
Tip: You can also try: spear, fire-magic, ice-magic, sword-magic, ring-of-fire, ring-of-ice, or ring-of-blades.
read
Code a game with a wood table crafter that slowly builds a high-damage sword.
code
#lang survival (define my-sword-recipe (recipe #:product (sword #:damage 100) #:build-time 100)) (survival-game #:crafter-list (list (basic-crafter #:sprite woodtable-sprite #:recipe-list (list my-sword-recipe))))
7.8 Weapon Crafter Kata (difficulty = 3)
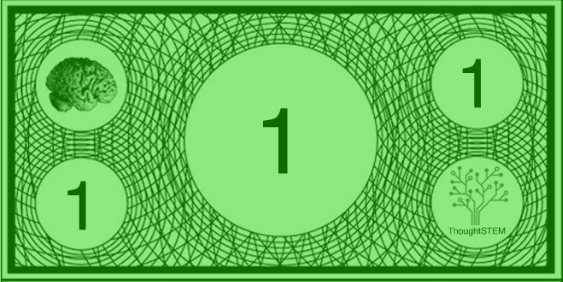
Tip: The default speed for fire-magic is 3.
read
Code a game with coins, and a wood table crafter that instantly builds fast-moving fire-magic for 100 gold.
code
#lang survival (define my-fire-magic-recipe (recipe #:product (fire-magic #:name "Fast Flame" #:speed 7) #:cost 100)) (survival-game #:coin-list (list (basic-coin)) #:crafter-list (list (basic-crafter #:sprite woodtable-sprite #:recipe-list (list my-fire-magic-recipe))))
7.9 Sky Kata (difficulty = 1)
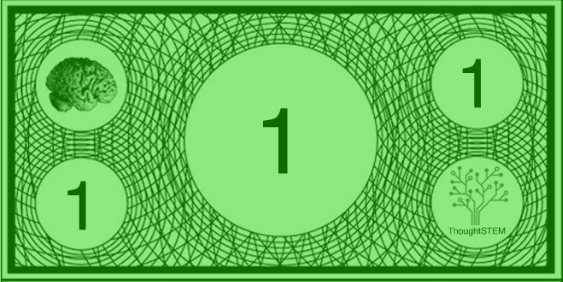
Tip: By default, the length-of-day will be 2400.
read
Code a game with a very long day/night cycle.
code
#lang survival (survival-game #:sky (basic-sky #:length-of-day 5000))
7.10 Sky Kata (difficulty = 1)
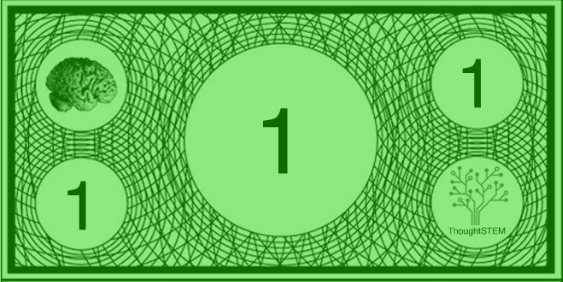
Tip: Max darkness takes a value between 0 (no darkness) and 255 (total blackout).
read
Code a game with a custom-colored night sky.
code
#lang survival (survival-game #:sky (basic-sky #:night-sky-color 'darkmagenta))
7.11 Sky Kata (difficulty = 1)
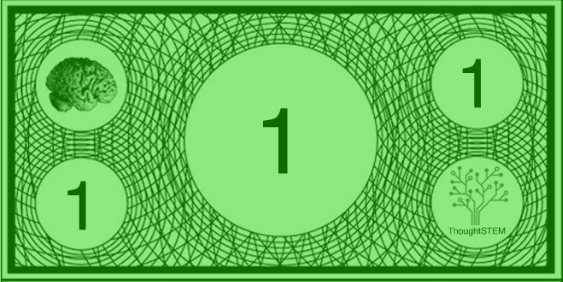
read
Code a game with a short day/night cycle where it gets pitch-black at midnight.
code
#lang survival (survival-game #:sky (basic-sky #:length-of-day 500 #:max-darkness 255))
7.12 Sky Kata (difficulty = 1)
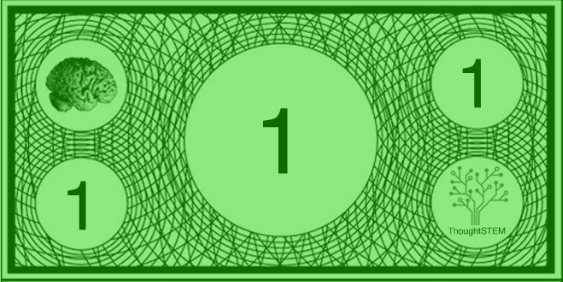
read
Code a game with a short night, and many nocturnal enemies.
code
#lang survival (survival-game #:enemy-list (list (basic-enemy #:amount-in-world 20 #:night-only? #t)) #:sky (basic-sky #:length-of-day 2400 #:start-of-daytime 200 #:end-of-daytime 2200))
7.13 Npc Kata (difficulty = 1)
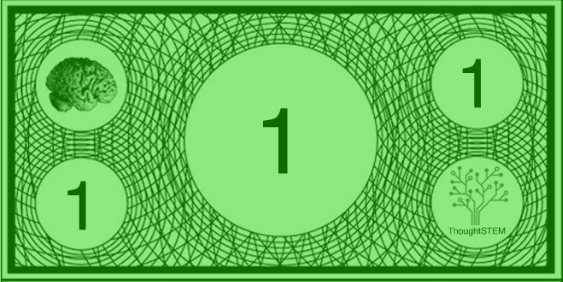
read
Code a game with a basic NPC.
code
#lang survival (survival-game #:npc-list (list (basic-npc)))
7.14 Npc Kata (difficulty = 2)
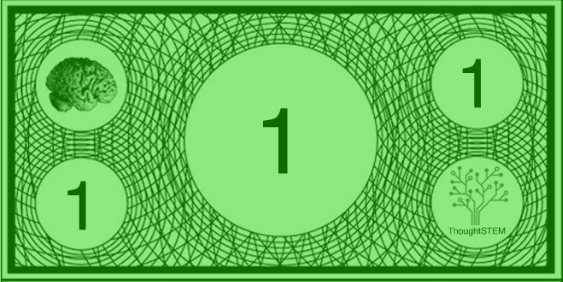
read
Code a game with a named witch NPC.
code
#lang survival (define (my-npc) (basic-npc #:sprite witch-sprite #:name "Witch")) (survival-game #:npc-list (list (my-npc)))
7.15 Npc Kata (difficulty = 2)
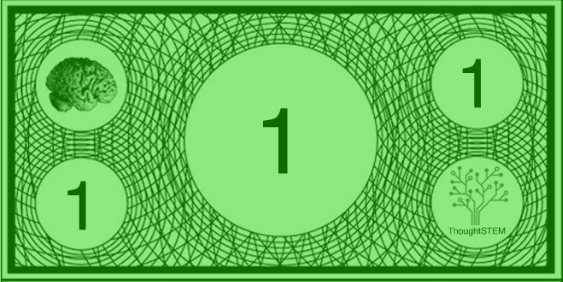
Tip: All options for #:mode are: ’follow, ’wander, ’pace and ’still.
read
Code a game with an NPC with custom dialog.
code
#lang survival (define (my-npc) (basic-npc #:dialog (list "Woah, who are you??" "Nevermind -- I'm too busy." "Move along, now!"))) (survival-game #:npc-list (list (my-npc)))
7.16 Npc Kata (difficulty = 2)
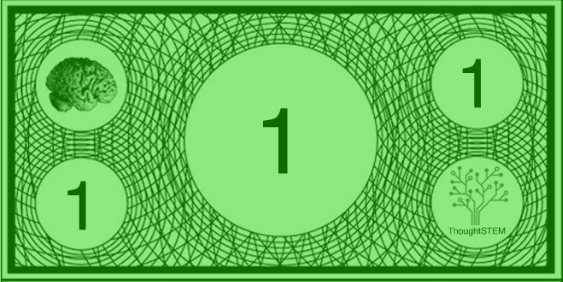
read
Code a game with a witch NPC who follows you. Give them a name and a specific start tile.
code
#lang survival (define (my-npc) (basic-npc #:sprite witch-sprite #:name "Witch" #:tile 3 #:mode 'follow)) (survival-game #:npc-list (list (my-npc)))
7.17 Npc Kata (difficulty = 4)
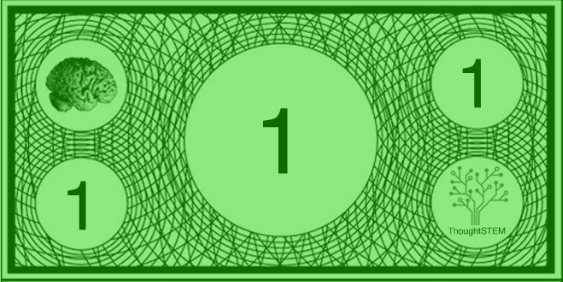
read
Code a game with 2 NPCs with custom dialog and two additional customizations each.
code
#lang survival (define (my-npc) (basic-npc #:name "Francis" #:tile 4 #:dialog (list "Greetings!" "You better find some food soon..."))) (define (another-npc) (basic-npc #:sprite witch-sprite #:mode 'pace #:dialog (list "Now where did I put it..." "Have you seen an eye of newt?" "Oh, I think I see it!"))) (survival-game #:npc-list (list (my-npc) (another-npc)))