3 Food Katas
3.1 Food Kata (difficulty = 3)
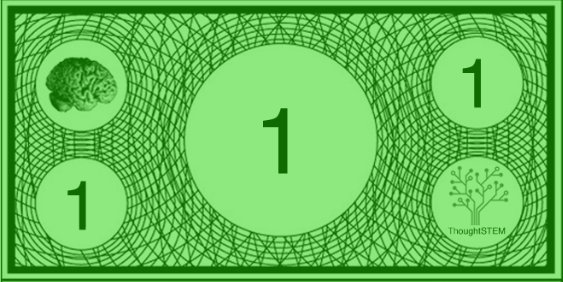
Tip: By default, basic-food will heal by 10.
read
Code a game with a couple of apples with extra healing power.
code
#lang survival-minecraft (define (my-food) (basic-food #:sprite apples-sprite #:name "Apples" #:amount-in-world 2 #:heals-by 20)) (minecraft-game #:food-list (list (my-food)))
3.2 Food Kata (difficulty = 6)
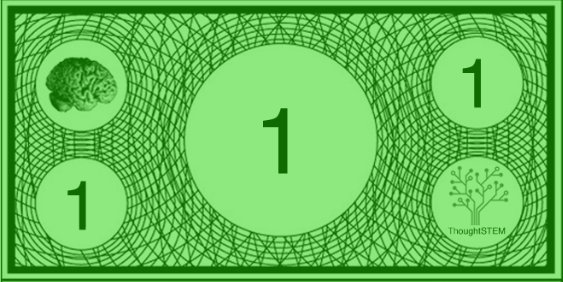
Tip: A very rare food might only ever spawn 1 time in a game.
read
Code a game with 2 different food types: common apples with low-healing power and one very rare cherry with high-healing power.
code
#lang survival-minecraft (define (my-food) (basic-food #:sprite apples-sprite #:name "Apples" #:amount-in-world 15 #:heals-by 5)) (define (special-food) (basic-food #:sprite cherry-sprite #:name "Cherry" #:amount-in-world 1 #:heals-by 50 #:respawn? #f)) (minecraft-game #:food-list (list (my-food) (special-food)))
3.3 Food Kata (difficulty = 3)
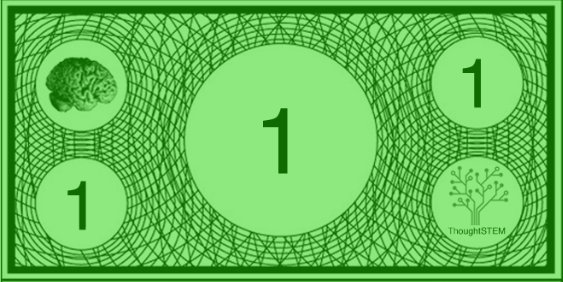
Tip: The default starvation rate is 50.
read
Code a game with a very fast starvation rate and lots of cherries with high-healing power.
code
#lang survival-minecraft (define (my-food) (basic-food #:sprite cherry-sprite #:name "Cherries" #:amount-in-world 20 #:heals-by 50)) (minecraft-game #:food-list (list (my-food)) #:starvation-rate 100)
3.4 Food Kata (difficulty = 8)
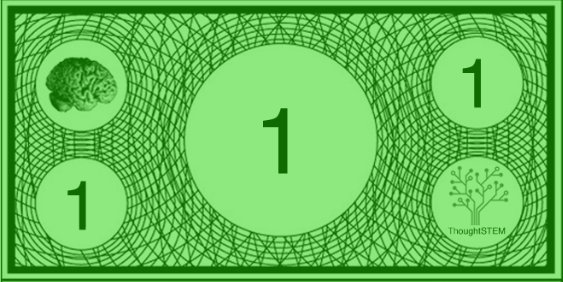
read
Code a game with 3 food types: common cherries, trick smores that deplete your health, and a single high-healing carrot that spawns, only once, on a specific tile.
code
#lang survival-minecraft (define (basic-cherry) (basic-food #:sprite cherry-sprite #:name "Cherries" #:amount-in-world 15)) (define (basic-smores) (basic-food #:sprite smores-sprite #:name "Smores" #:heals-by -10)) (define (special-carrot) (basic-food #:sprite carrot-sprite #:name "Carrots" #:tile 4 #:amount-in-world 1 #:heals-by 50 #:respawn? #f)) (minecraft-game #:food-list (list (basic-cherry) (basic-smores) (special-carrot)))
3.5 Crafter Kata (difficulty = 6)
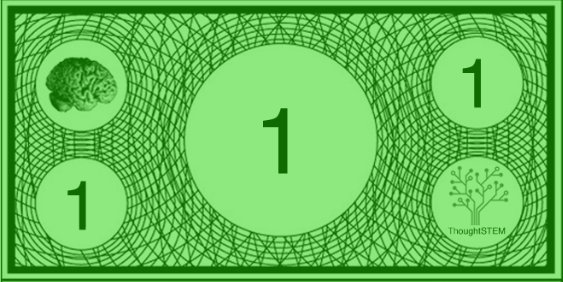
Tip: Don’t forget the recipe.
read
Code a game with fish, and a crafter that makes fish stew with high-healing power.
code
#lang survival-minecraft (define (fish-stew) (basic-product #:name "Fish Stew" #:sprite fishstew-sprite #:heals-by 50)) (define fish-stew-recipe (recipe #:product (fish-stew) #:ingredients (list "Fish"))) (minecraft-game #:food-list (list (fish)) #:crafter-list (list (basic-crafter #:recipe-list (list fish-stew-recipe))))