4 Mob & Entity Katas
4.1 Mob Kata (difficulty = 3)
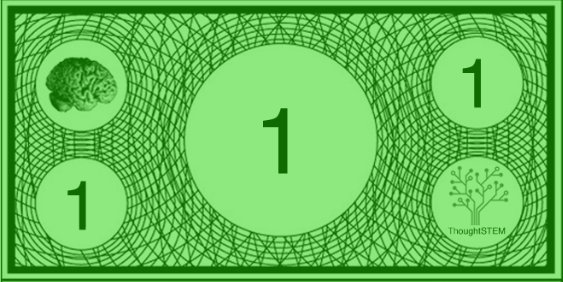
Tip: The options for #:ai are ’easy, ’medium and ’hard.
read
Code a game with 5 moderately intelligent skeleton mobs.
code
#lang survival-minecraft (define (my-mob) (basic-mob #:ai 'medium #:sprite skeleton-sprite #:amount-in-world 5)) (minecraft-game #:mob-list (list (my-mob)))
4.2 Mob Kata (difficulty = 5)
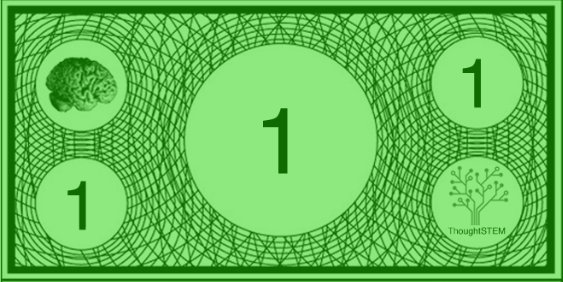
read
Code a game with 10 total mobs: half are creepers with low intelligence, and half are moderately intelligent, nocturnal skeletons.
code
#lang survival-minecraft (define (easy-mob) (basic-mob #:ai 'easy #:sprite creeper-sprite #:amount-in-world 5)) (define (medium-mob) (basic-mob #:ai 'medium #:sprite skeleton-sprite #:amount-in-world 5 #:night-only? #t)) (minecraft-game #:mob-list (list (easy-mob) (medium-mob)))
4.3 Mob Kata (difficulty = 6)
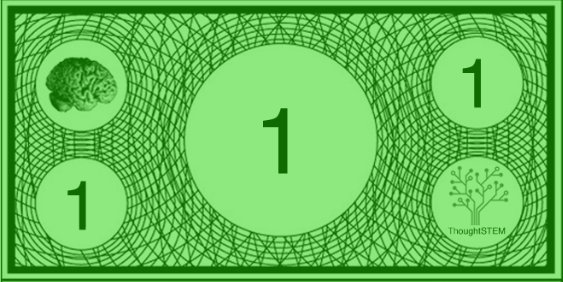
Tip: Ghasts shoot fireballs.
read
Code a game with 2 types of mobs: a few moderately intelligent skeletons, and several highly intelligent, nocturnal ghasts with a high-damage attack.
code
#lang survival-minecraft (define (medium-mob) (basic-mob #:ai 'medium #:sprite skeleton-sprite #:amount-in-world 3)) (define (hard-mob) (basic-mob #:ai 'hard #:sprite ghast-sprite #:amount-in-world 5 #:night-only? #t #:weapon (fireball #:damage 50))) (minecraft-game #:mob-list (list (medium-mob) (hard-mob)))
4.4 Entity Kata (difficulty = 3)
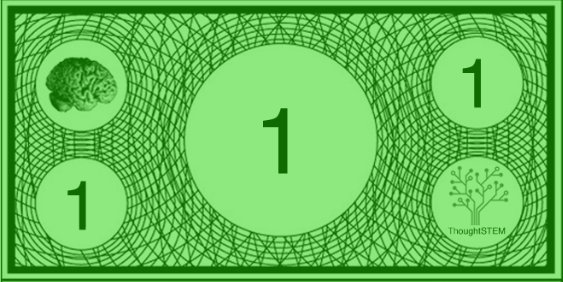
Tip: All options for #:mode are: ’follow, ’wander, ’pace and ’still.
read
Code a game with a pig entity who follows you. Give them a name and a specific tile location.
code
#lang survival-minecraft (define (my-entity) (basic-entity #:sprite pig-sprite #:name "Sir Pigsnoot" #:tile 3 #:mode 'follow)) (minecraft-game #:entity-list (list (my-entity)))
4.5 Entity Kata (difficulty = 6)
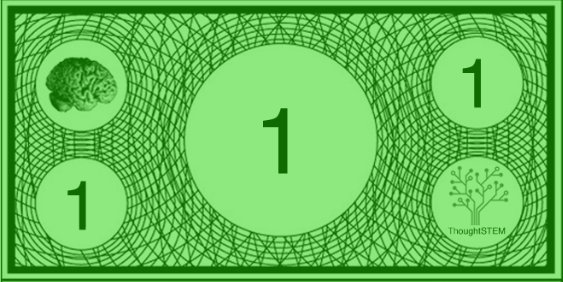
Tip: The customization options for npcs are: #:sprite, #:position, #:name, #:tile, #:mode, #:game-width, #:speed, #:target, #:sound, #:scale, and #:components.
read
Code a game with 2 NPCs with custom dialog and two additional customizations each.
code
#lang survival-minecraft (define (my-entity) (basic-entity #:name "Francis" #:tile 4 #:dialog (list "Greetings!" "Gee, you look hungry."))) (define (another-entity) (basic-entity #:sprite chicken-sprite #:name "Mr. Chick Chickenson III" #:mode 'pace #:dialog (list "Woah, who are you??" "Wait, I'm a chicken..." "I can't talk!"))) (minecraft-game #:entity-list (list (my-entity) (another-entity)))