5 Ore & Weapons Katas
5.1 Ore Kata (difficulty = 1)
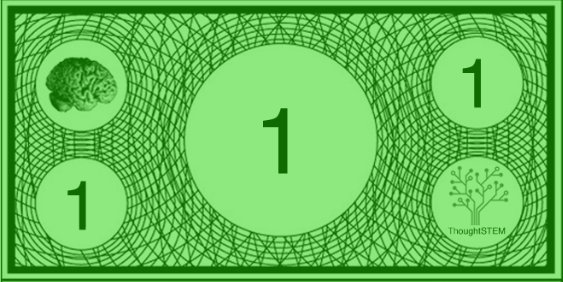
Tip: The default value of basic-ore is 10.
read
Code a game with valuable ore.
code
#lang survival-minecraft (minecraft-game #:ore-list (list (basic-ore #:value 50)))
5.2 Ore Kata (difficulty = 3)
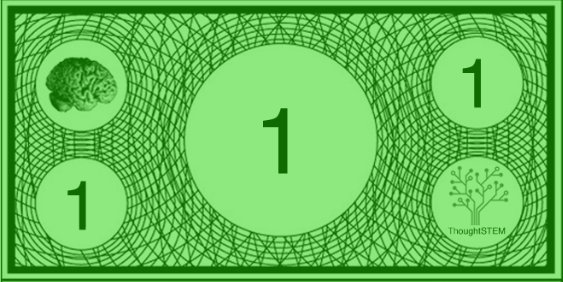
read
Code a game with many valuable gold ore blocks.
code
#lang survival-minecraft (define (my-ore) (basic-ore #:sprite goldore-sprite #:name "Gold Ore" #:value 200 #:amount-in-world 20)) (minecraft-game #:ore-list (list (my-ore)))
5.3 Ore Kata (difficulty = 5)
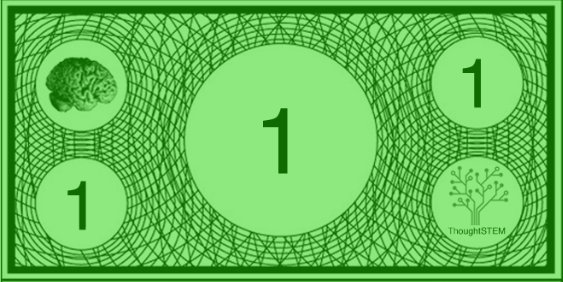
read
Code a game with gold ore and an extremely rare and valuable diamond.
code
#lang survival-minecraft (define (gold-ore) (basic-ore #:sprite goldore-sprite #:name "Gold Ore")) (define (diamond) (basic-ore #:sprite diamond-sprite #:name "Diamond" #:value 1000 #:amount-in-world 1 #:respawn? #f)) (minecraft-game #:ore-list (list (gold-ore) (diamond)))
5.4 Weapon Crafter Kata (difficulty = 4)
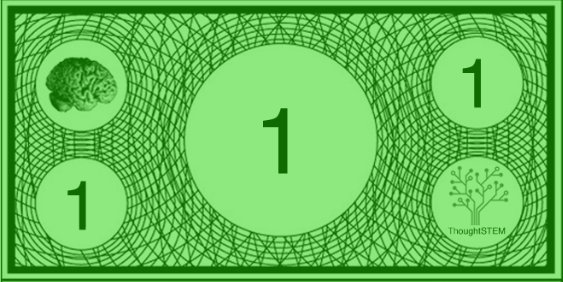
Tip: You can also try: spear, fire-magic, ice-magic, sword-magic, ring-of-fire, ring-of-ice, or ring-of-blades.
read
Code a game with a wood table crafter that slowly builds a high-damage sword.
code
#lang survival-minecraft (define my-sword-recipe (recipe #:product (sword #:damage 100) #:build-time 100)) (minecraft-game #:crafter-list (list (basic-crafter #:sprite woodtable-sprite #:recipe-list (list my-sword-recipe))))
5.5 Weapon Crafter Kata (difficulty = 5)
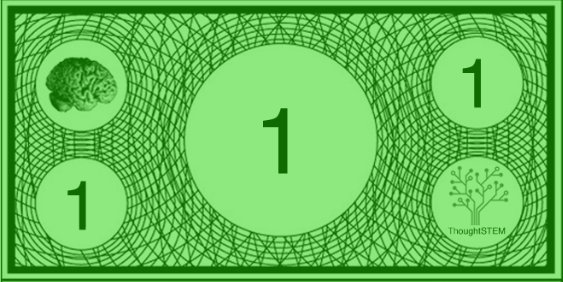
Tip: The default speed for fire-magic is 3.
read
Code a game with ore, and a wood table crafter that instantly builds fast-moving fire magic for 100 ore.
code
#lang survival-minecraft (define my-fire-magic-recipe (recipe #:product (fire-magic #:name "Fast Flame" #:speed 7) #:cost 100)) (minecraft-game #:ore-list (list (basic-ore)) #:crafter-list (list (basic-crafter #:sprite woodtable-sprite #:recipe-list (list my-fire-magic-recipe))))