4 Moon And Ring Katas
4.1 Moon And Ring 1 Kata (difficulty = 2)
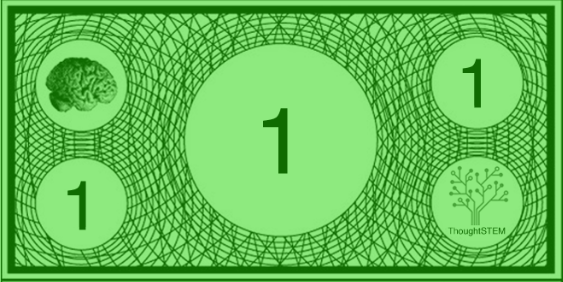
read
Code an orbit scene with a star center and a planet with a moon.
code
#lang 3d-orbit (define planet-with-moon (basic-planet #:moons-list (list (basic-moon)))) (orbit-scene #:star (basic-star #:planets-list (list planet-with-moon)))
4.2 Moon And Ring 2 Kata (difficulty = 3)
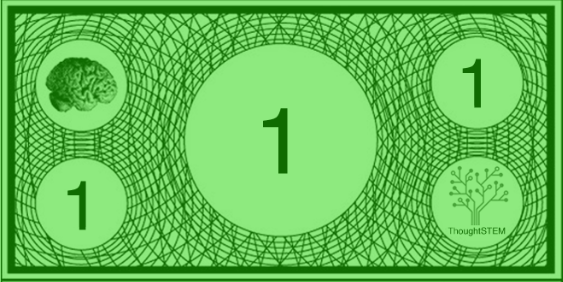
Tip: When the show-orbits? keyword is set to #t it will add orbit tracks to the objects.
read
Code an orbit scene with two moons, a planet, and a star. The moons and the planet should have a visible orbit path.
code
#lang 3d-orbit (define my-moons (list (basic-moon) (basic-moon))) (define the-planet (basic-planet #:show-orbits? #t #:moons-list my-moons)) (orbit-scene #:star (basic-star #:show-orbits? #t #:planets-list (list the-planet)))
4.3 Moon And Ring 3 Kata (difficulty = 4)
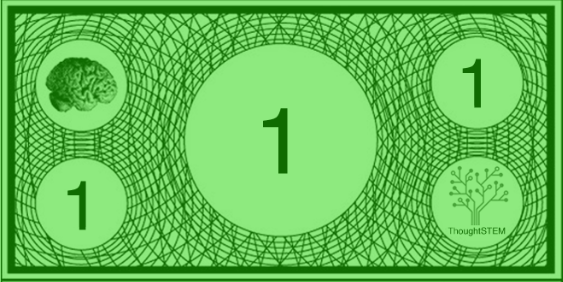
Tip: You can pass multiple changes to the on-mouse-enter and on-mouse-leave keywords using do-many.
read
Code an orbit scene with a star center and a planet with two moons. Show the orbit paths for the moons and planet.
code
#lang 3d-orbit (define my-moons (list (basic-moon #:texture sun-tex #:scale 2) (basic-moon #:label "This moon grows!" #:on-mouse-enter (do-many (scale 2)) #:on-mouse-leave (do-many (scale 1))))) (orbit-scene #:star (basic-star #:animations-list '() #:planets-list (list (basic-planet #:moons-list my-moons))))
4.4 Moon And Ring 4 Kata (difficulty = 2)
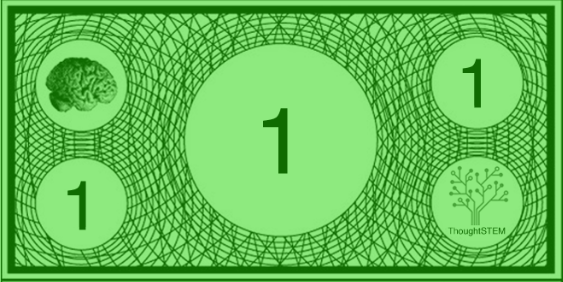
Tip: The default radius for the basic ring is a random number between 0.25 and 1.5 plus the radius of the planet.
read
Code an orbit scene with a star center and a ringed planet.
code
#lang 3d-orbit (define planet-with-ring (basic-planet #:rings-list (list (basic-ring)))) (orbit-scene #:star (basic-star #:planets-list (list planet-with-ring)))
4.5 Moon And Ring 5 Kata (difficulty = 3)
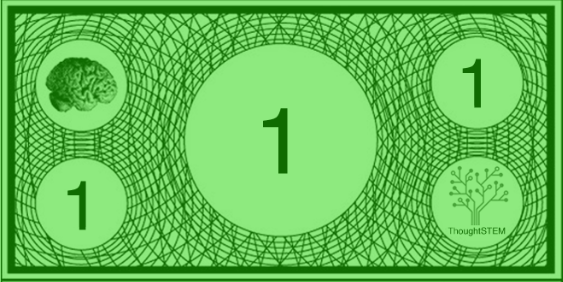
Tip: The default tilt for the basic ring is (0 0 0).
read
Code an orbit scene with a star center and a planet with two rings. Customize the color and opacity of one ring and the texture and tilt of the other ring.
code
#lang 3d-orbit (define my-rings (list (basic-ring #:color 'red #:opacity 0.8) (basic-ring #:texture saturnring-tex #:tilt (tilt 90 0 90)))) (orbit-scene #:star (basic-star #:planets-list (list (basic-planet #:rings-list my-rings))))