5 Space Objects Katas
5.1 Space Objects 1 Kata (difficulty = 2)
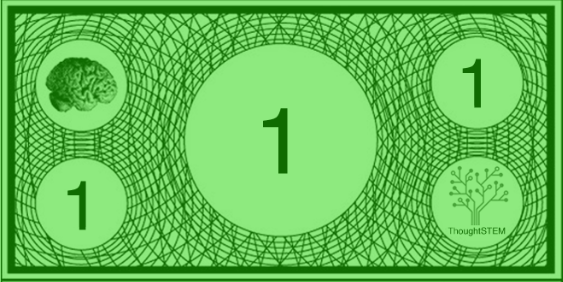
read
Code an orbit scene with a star center. Add three asteroids and customize each.
code
#lang 3d-orbit (define asteroids-list (list (basic-asteroid #:color 'red) (basic-asteroid #:opacity 0.7) (basic-asteroid #:label "Big Rock"))) (orbit-scene #:star (basic-star) #:objects-list asteroids-list)
5.2 Space Objects 2 Kata (difficulty = 3)
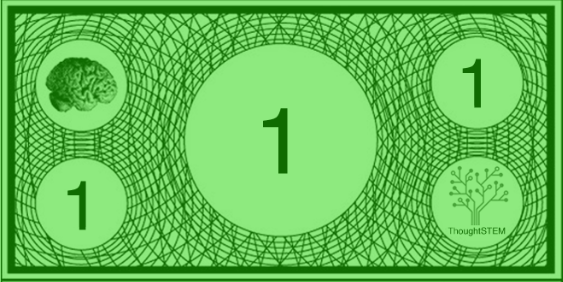
Tip: The default radius for the basic asteroid is a random number between 0.1 and 0.3 plus the radius of the planet.
read
Code an orbit scene with a star center and three orbiting asteroids. Customize each asteroid.
code
#lang 3d-orbit (define asteroids-list (list (basic-asteroid #:texture mars-tex) (basic-asteroid #:radius 0.5) (basic-asteroid #:on-mouse-click (do-many (opacity 0))))) (orbit-scene #:star (basic-star #:objects-list asteroids-list))
5.3 Space Objects 3 Kata (difficulty = 2)
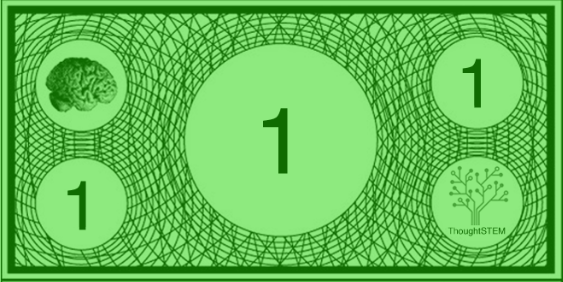
read
Code an orbit scene with a star center and a planet. Give the planet three orbiting objects: a moon, an asteroid, and an astronaut.
code
#lang 3d-orbit (define my-planet (basic-planet #:objects-list (list (basic-moon) (basic-asteroid) astronaut))) (orbit-scene #:star (basic-star #:planets-list (list my-planet)))
5.4 Space Objects 4 Kata (difficulty = 2)
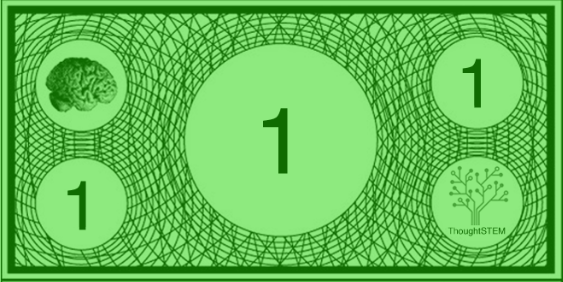
Tip: If you want to use scale-object with a celestial object make sure to pass it as a function; e.g. (basic-star).
read
Code an orbit scene with a star center and a planet. Give the planet a small satellite.
code
#lang 3d-orbit (define my-planet (basic-planet #:objects-list (list (scale-object 0.5 satellite-1)))) (orbit-scene #:star (basic-star #:planets-list (list my-planet)))
5.5 Space Objects 5 Kata (difficulty = 3)
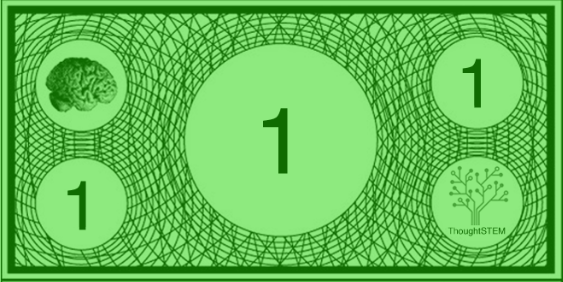
read
Code an orbit scene with a star center which has an orbiting planet, which has an orbiting moon, which has an orbiting flying saucer.
code
#lang 3d-orbit (define my-moon (basic-moon #:objects-list (list flying-saucer-1))) (define my-planet (basic-planet #:moons-list (list my-moon))) (orbit-scene #:star (basic-star #:planets-list (list my-planet)))