6 Star System Katas
6.1 Star System 1 Kata (difficulty = 3)
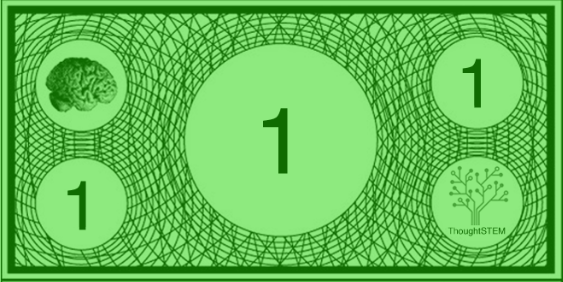
Tip: If you run (planets-to-scale) you will see the sun and all the planets aligned next to each other.
read
Code an orbit scene with the Sun and our first 3 planets. Use the appropriate textures, add labels, and give Earth a moon.
code
#lang 3d-orbit (define the-moon (basic-moon #:texture moon-tex #:label "Luna")) (define my-planets (list (basic-planet #:texture mercury-tex #:label "Mercury") (basic-planet #:texture venus-tex #:label "Venus") (basic-planet #:texture earth-tex #:label "Earth" #:moons-list (list the-moon)))) (orbit-scene #:star (basic-star #:texture sun-tex #:label "Sol" #:planets-list my-planets))
6.2 Star System 2 Kata (difficulty = 3)
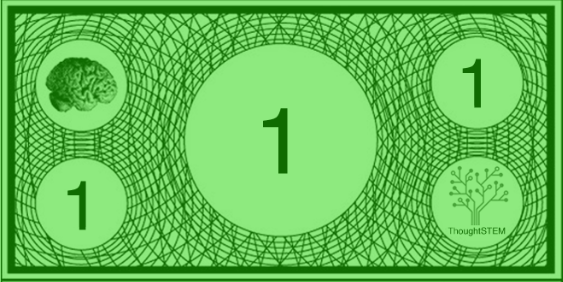
Tip: If you run (solar-system) you will see how far the planets are from the sun. Although, in reality the planets are 100x smaller and the sun is 10x bigger.
read
Code an orbit scene with the Sun, Jupiter, and Saturn. Use the appropriate textures, add labels, and give Saturn a ring.
code
#lang 3d-orbit (define the-ring (basic-ring #:tilt (tilt 90 0 0) #:texture saturnring-tex #:radius 2 #:thickness 0.5)) (define my-planets (list (basic-planet #:texture jupiter-tex #:label "Jupiter") (basic-planet #:texture saturn-tex #:label "Saturn" #:rings-list (list the-ring)))) (orbit-scene #:star (basic-star #:texture sun-tex #:label "Sol" #:planets-list my-planets))
6.3 Star System 3 Kata (difficulty = 2)
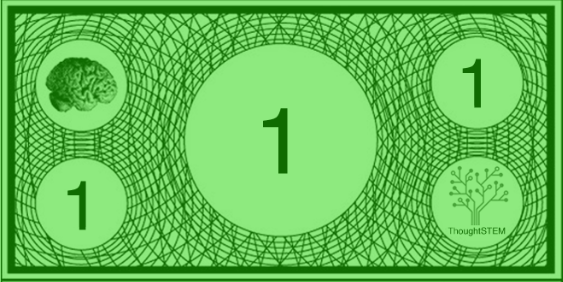
Tip: If you run (stars-to-scale) you will see several stars, including the sun, next to each other.
read
Code an orbit scene with a unique star system. Add at least 2 custom planets with custom textures and names.
code
#lang 3d-orbit (define my-planets (list (basic-planet #:texture brick-tex #:label "Brick World") (basic-planet #:texture steel-tex #:label "Steel World"))) (orbit-scene #:star (basic-star #:planets-list my-planets))
6.4 Star System 4 Kata (difficulty = 3)
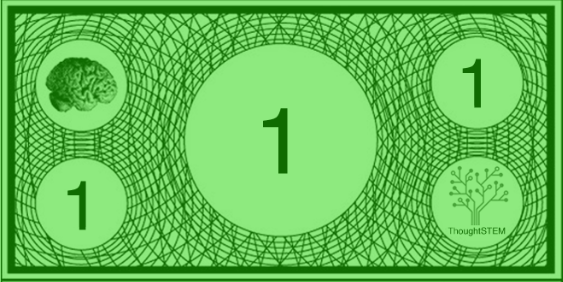
read
Code an orbit scene with a unique star system. Add at least 2 custom planets with moons and/or rings.
code
#lang 3d-orbit (define moon-planet (basic-planet #:moons-list (list (basic-moon #:texture black-tex)))) (define ring-planet (basic-planet #:rings-list (list (basic-ring #:texture pink-tex)))) (orbit-scene #:star (basic-star #:planets-list (list moon-planet ring-planet)))
6.5 Star System 5 Kata (difficulty = 2)
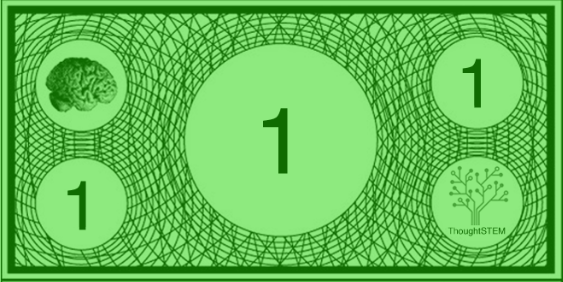
read
Code an orbit scene with a unique star system. Add at least 2 custom planets and 3 space objects.
code
#lang 3d-orbit (define my-planet (basic-planet #:texture purple-tex)) (orbit-scene #:star (basic-star #:planets-list (list my-planet my-planet)) #:objects-list (list international-space-station space-shuttle asteroids))