3 Star And Planet Katas
3.1 Star And Planet 1 Kata (difficulty = 1)
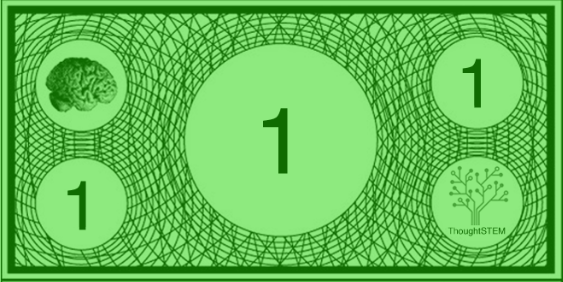
read
Code an orbit scene with a planet rotating around a star center.
code
#lang 3d-orbit (orbit-scene #:star (basic-star #:planets-list (list (basic-planet))))
3.2 Star And Planet 2 Kata (difficulty = 2)
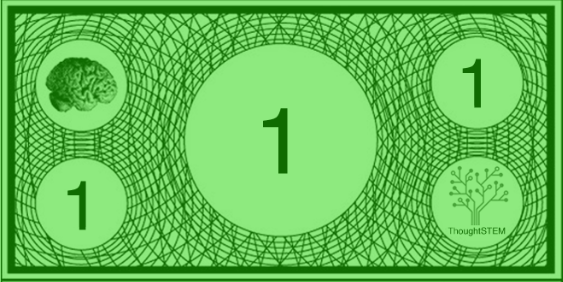
Tip: The default radius for a planet is a random number between 1 and 5.
read
Code an orbit scene with a planet rotating around a star center. Customize the radius, opacity, and color of the planet and star.
code
#lang 3d-orbit (define my-planet (basic-planet #:radius 5 #:opacity 0.8 #:color 'blue)) (define my-star (basic-star #:radius 5 #:opacity 0.8 #:color 'yellow #:planets-list (list my-planet))) (orbit-scene #:star my-star)
3.3 Star And Planet 3 Kata (difficulty = 3)
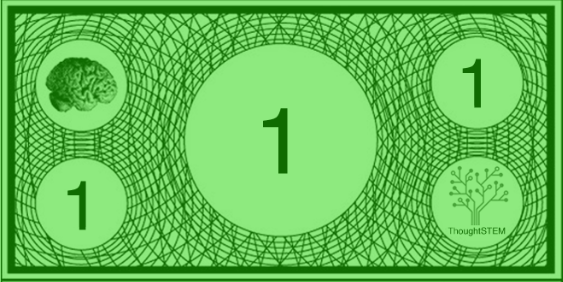
read
Code an orbit scene with a star center. Change the star’s opacity when clicked. Add a planet whose texture changes when you look directly at it.
code
#lang 3d-orbit (define changing-planet (basic-planet #:texture jupiter-tex #:on-mouse-enter (do-many (texture mars-tex)) #:on-mouse-leave (do-many (texture jupiter-tex)))) (orbit-scene #:star (basic-star #:texture sun-tex #:on-mouse-click (do-many (opacity 0.1)) #:planets-list (list changing-planet)))
3.4 Star And Planet 4 Kata (difficulty = 2)
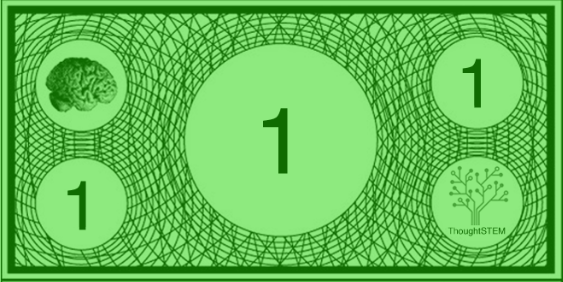
Tip: The default rotation for a basic planet is on the x-axis. If animations gets an empty list the object will not rotate.
read
Code an orbit scene with a non-moving Sun center. Add an Earth planet which rotates on the y-axis. Add a custom label to both.
code
#lang 3d-orbit (define my-planet (basic-planet #:label "Earth" #:label-color 'blue #:texture earth-tex #:animations-list (list (y-rotation)))) (orbit-scene #:star (basic-star #:animations-list '() #:label "Sol" #:label-color 'orange #:texture sun-tex #:planets-list (list my-planet)))
3.5 Star And Planet 5 Kata (difficulty = 2)
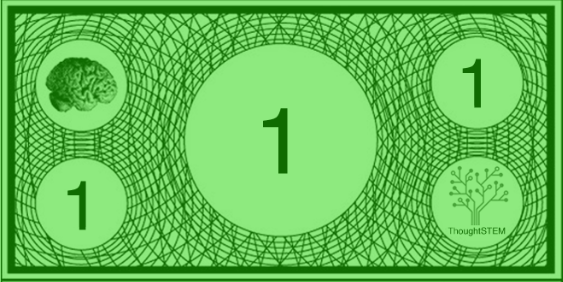
Tip: Earth is actually 100x further from the Sun, the position (23000 0 0) would be more accurate.
read
Code an orbit scene with Earth and Sun to scale. Customize Earth’s position and show the orbit path.
code
#lang 3d-orbit (define the-earth (planet-earth #:position (position 230 0 0))) (orbit-scene #:star (star-sun #:show-orbits? #t #:planets-list (list the-earth)))