3 Enemy Katas
3.1 Enemy 1 Kata (difficulty = 1)
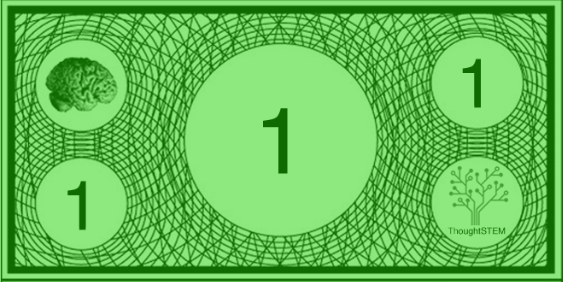
read
Code a game with 10 strong, nocturnal enemies with moderate intelligence and each with a random sprite.
code
#lang adventure (adventure-game #:enemy-list (list (curry basic-enemy #:ai 'medium #:amount-in-world 10 #:night-only? #t #:health 200)))
3.2 Enemy 2 Kata (difficulty = 4)
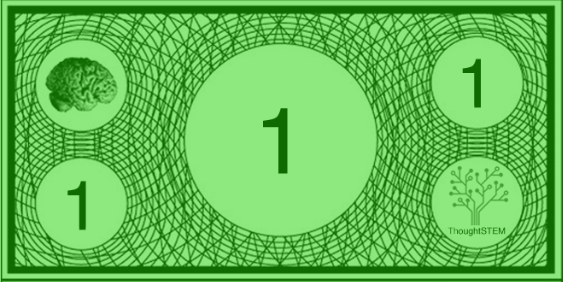
read
Code a game with several enemies: 4 low intelligence slime, 2 moderately intelligent bats, and a highly intelligent, nocturnal snake.
code
#lang adventure (define (easy-enemy) (basic-enemy #:ai 'easy #:sprite slime-sprite #:amount-in-world 4)) (define (medium-enemy) (basic-enemy #:ai 'medium #:sprite bat-sprite #:amount-in-world 2)) (define (hard-enemy) (basic-enemy #:ai 'hard #:sprite snake-sprite #:night-only? #t)) (adventure-game #:enemy-list (list (easy-enemy) (medium-enemy) (hard-enemy)))
3.3 Enemy 3 Kata (difficulty = 4)
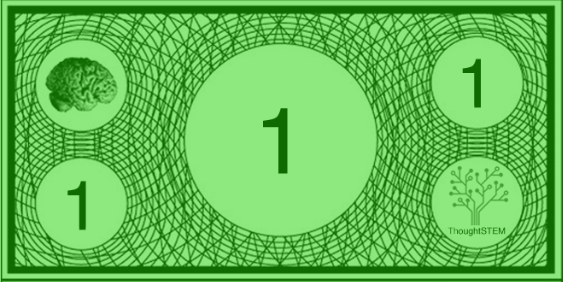
read
Code a game with a spear and 3 highly intelligent enemies that drop valuable gold coins.
code
#lang adventure (define (my-gold-coin) (basic-coin #:sprite goldcoin-sprite #:value 20)) (define (hard-enemy) (basic-enemy #:amount-in-world 3 #:ai 'hard #:loot-list (list (my-gold-coin) (my-gold-coin)))) (adventure-game #:weapon-list (list (spear)) #:enemy-list (list (hard-enemy)))
3.4 Enemy 4 Kata (difficulty = 5)
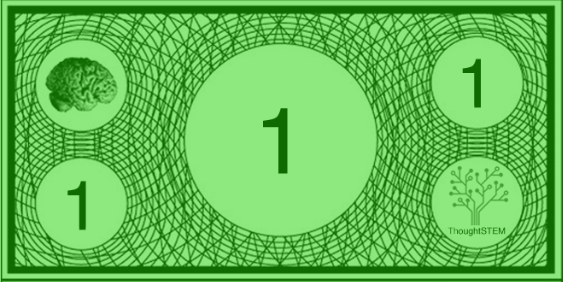
read
Code a game with an avatar, a spear, and 3 highly intelligent enemies that shoot fireballs. Include a creative game over cutscene.
code
#lang adventure (define (hard-enemy) (basic-enemy #:amount-in-world 3 #:ai 'hard #:weapon (fireball))) (adventure-game #:avatar (basic-avatar #:sprite steampunkboy-sprite) #:death-cutscene (basic-cutscene (page (set-sprite-angle 90 (render steampunkboy-sprite)) "You died!") (page "Try harder!")) #:weapon-list (list (spear)) #:enemy-list (list (hard-enemy)))
3.5 Enemy 5 Kata (difficulty = 4)
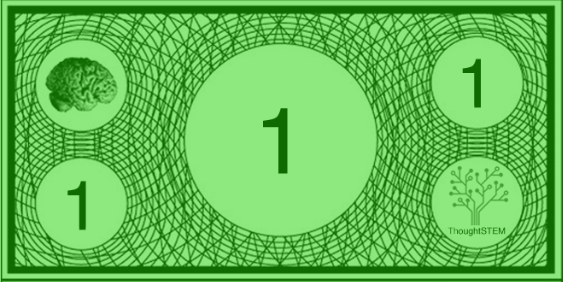
read
Code a game with an NPC who has a quest to slay several enemies; the quest has a point and item reward. Include 10 random enemies, a fireball power, and a game over cutscene.
code
#lang adventure (define my-hunt-quest (hunt-quest #:hunt-amount 5 #:reward-amount 50 #:reward-item (sword))) (adventure-game #:death-cutscene (basic-cutscene (page "You died!" "Try harder!")) #:npc-list (list (basic-npc #:dialog (list "These monsters are annoying." "Can you get rid of 5?") #:quest-list (list my-hunt-quest))) #:weapon-list (list (fireball)) #:enemy-list (list (curry basic-enemy #:amount-in-world 10)))