7 Extra Katas
7.1 Level Design 1 Kata (difficulty = 1)
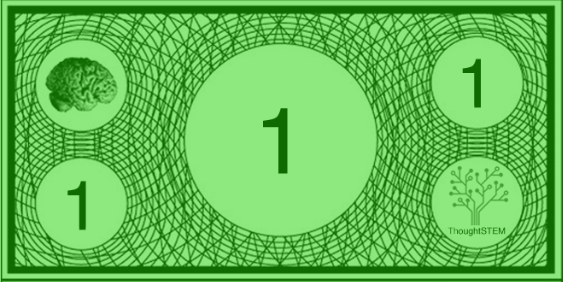
read
Code a game with a forest level that has world objects.
code
#lang adventure (adventure-game #:bg (basic-bg #:image FOREST-BG) #:enable-world-objects? #t)
7.2 Level Design 2 Kata (difficulty = 1)
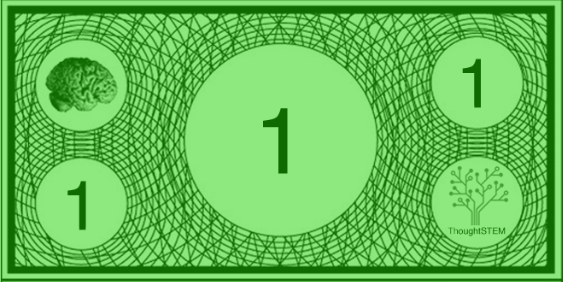
read
Code a game with a candy level and 2 types of randomly-colored, high-definition trees.
code
#lang adventure (adventure-game #:bg (basic-bg #:image PINK-BG) #:other-entities (make-world-objects candy-cane-tree snow-pine-tree #:hd? #t #:random-color? #t))
7.3 Level Design 3 Kata (difficulty = 1)
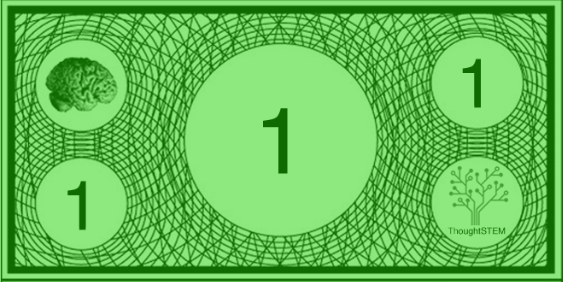
read
Code a game with a desert level filled with random brown rocks. Make everything high-definition.
code
#lang adventure (adventure-game #:bg (basic-bg #:image DESERT-BG #:hd? #t) #:other-entities (make-world-objects random-brown-rock random-brown-rock #:hd? #t))
7.4 Level Design 4 Kata (difficulty = 2)
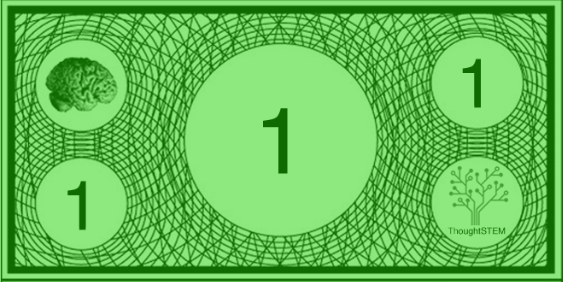
read
Code a game with a lava level filled with high-definition gray rocks. Add an intro cutscene with three pages.
code
#lang adventure (adventure-game #:bg (basic-bg #:image LAVA-BG #:hd? #t) #:intro-cutscene (basic-cutscene (page "Once upon a time" "on a volcanic moon" "of a distance world...") (page "There was a lone soldier" "who was banished from his" "homeworld.") (page (set-sprite-scale 2 basic-sprite) "This is his story.")) #:other-entities (make-world-objects random-gray-rock random-gray-rock #:hd? #t))
7.5 Level Design 5 Kata (difficulty = 3)
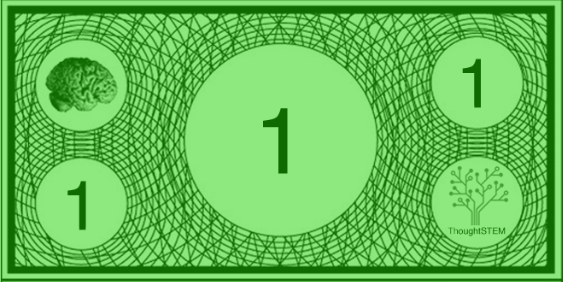
read
Code a game with a big gray rock, a randomly-colored brick house, and low-quality barrels. Manually set positions and tiles of all these entities.
code
#lang adventure (adventure-game #:bg (basic-bg) #:avatar (basic-avatar) #:other-entities (reduce-quality-by 2 (barrels (posn 100 200) #:tile 0)) (large-gray-rock (posn 100 200) #:tile 1 #:size 2) (brick-house (posn 200 200) #:tile 2 #:hue (random 360)))
7.6 Coin 1 Kata (difficulty = 1)
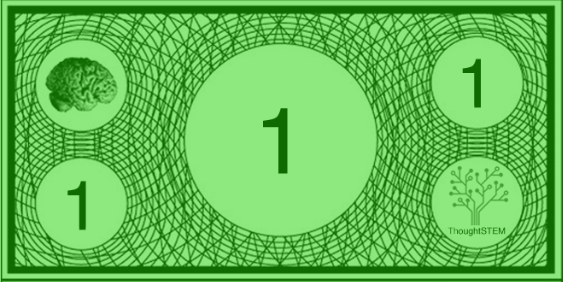
read
Code a game with 20 vaulable gold coins that do not respawn.
code
#lang adventure (adventure-game #:coin-list (list (basic-coin #:sprite goldcoin-sprite #:value 100 #:amount-in-world 20 #:respawn? #f)))
7.7 Coin 2 Kata (difficulty = 4)
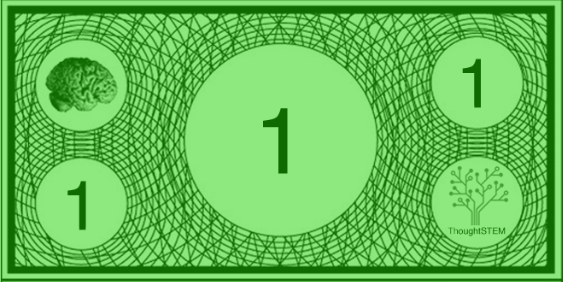
read
Code a game with cheap copper coins, more valuable silver coins, and very valuable gold coins.
code
#lang adventure (define (copper-coin) (basic-coin #:sprite coppercoin-sprite #:name "Copper Coin" #:value 1)) (define (silver-coin) (basic-coin #:sprite silvercoin-sprite #:name "Silver Coin" #:value 25)) (define (gold-coin) (basic-coin #:sprite goldcoin-sprite #:name "Gold Coin" #:value 50)) (adventure-game #:coin-list (list (copper-coin) (silver-coin) (gold-coin)))
7.8 Coin 3 Kata (difficulty = 3)
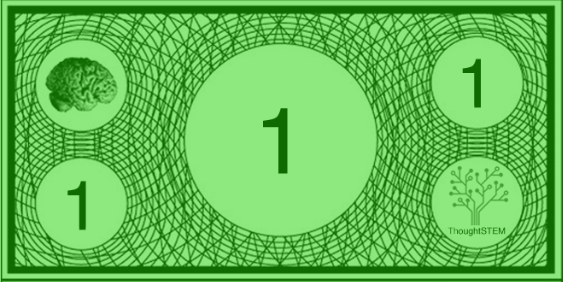
read
Code a game with a very rare, trick gold coin. Make the coin worth -1000 and spawn an enemy when picked up.
code
#lang adventure (define (my-trick-coin) (basic-coin #:name "Gold Coin" #:sprite goldcoin-sprite #:value -1000 #:amount-in-world 1 #:respawn? #f #:on-pickup (spawn-on-current-tile (basic-enemy #:position (posn 0 0) #:tile 0)))) (adventure-game #:coin-list (list (my-trick-coin)))
7.9 Coin 4 Kata (difficulty = 2)
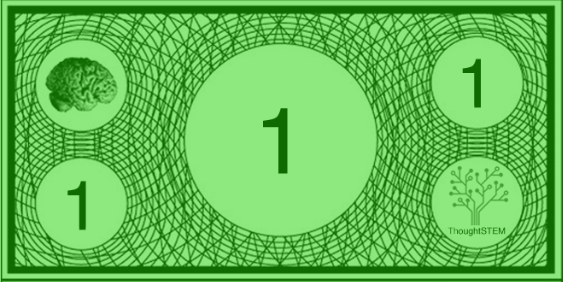
read
Code a game with an extremely rare and valuable gold coin. When the coin is picked up, spawn a cutscene with a gold coin and some text.
code
#lang adventure (define (my-special-coin) (basic-coin #:name "Gold Coin" #:sprite goldcoin-sprite #:value 1000 #:amount-in-world 1 #:respawn? #f #:on-pickup (spawn (page goldcoin-sprite "This looks valuable.")))) (adventure-game #:coin-list (list (my-special-coin)))
7.10 Coin 5 Kata (difficulty = 4)
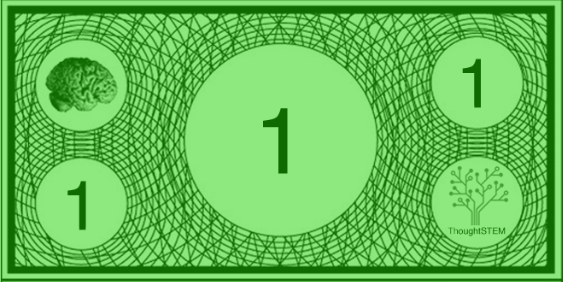
read
Code a game with 15 coins that do not respawn and an NPC. Give a collect quest where you have to collect 100 points for a reward.
code
#lang adventure (define (my-coin) (basic-coin #:amount-in-world 15 #:respawn? #f)) (define (npc-with-coin-quest) (basic-npc #:dialog (list "I've lost my coins..." "...100 points worth!" "Can you find them for me?") #:quest-list (list (collect-quest #:collect-amount 100 #:reward-amount 50)))) (adventure-game #:npc-list (list (npc-with-coin-quest)) #:coin-list (list (my-coin)))
7.11 Crafter 1 Kata (difficulty = 1)
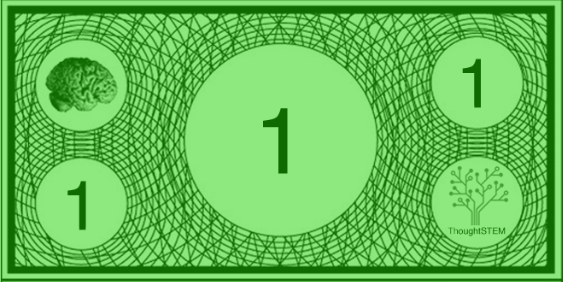
read
Code a game with an avatar and a crafter.
code
#lang adventure (adventure-game #:avatar (basic-avatar) #:crafter-list (list (basic-crafter)))
7.12 Crafter 2 Kata (difficulty = 2)
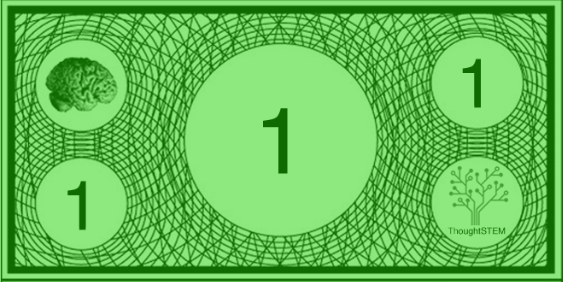
read
Code a game with 10 carrots and a crafter that produces carrot stew.
code
#lang adventure (adventure-game #:avatar (basic-avatar) #:food-list (list (carrot #:amount-in-world 10)) #:crafter-list (list (basic-crafter #:recipe-list (list carrot-stew-recipe))))
7.13 Crafter 3 Kata (difficulty = 4)
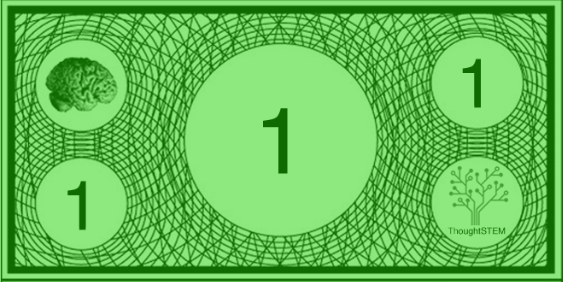
read
Code a game with a 10 fish, and a fish stew recipe, and a cauldron that produces fish stew.
code
#lang adventure (define (fish-stew) (basic-food #:name "Fish Stew" #:sprite fishstew-sprite #:respawn? #f #:heals-by 50)) (define fish-stew-recipe (recipe #:product (fish-stew) #:build-time 40 #:ingredients (list "Fish"))) (adventure-game #:avatar (basic-avatar) #:food-list (list (fish #:amount-in-world 10)) #:crafter-list (list (basic-crafter #:sprite cauldron-sprite #:recipe-list (list fish-stew-recipe))))
7.14 Crafter 4 Kata (difficulty = 6)
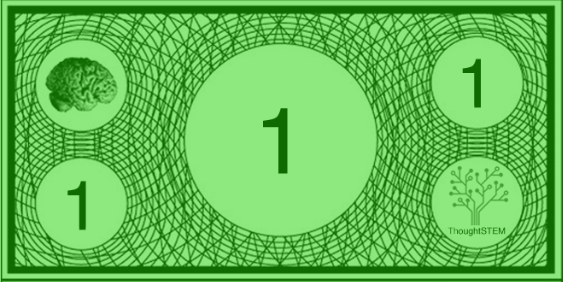
read
Code a game with 10 fish,10 carrots, a fish stew recipe, and a cauldron that produces fish stew and carrot stew. Customize the tile and position of the cauldron.
code
#lang adventure (define (fish-stew) (basic-food #:name "Fish Stew" #:sprite fishstew-sprite #:respawn? #f #:heals-by 50)) (define fish-stew-recipe (recipe #:product (fish-stew) #:build-time 40 #:ingredients (list "Fish"))) (define (my-cauldron) (basic-crafter #:sprite cauldron-sprite #:position (posn 200 200) #:tile 2 #:recipe-list (list carrot-stew-recipe fish-stew-recipe))) (adventure-game #:avatar (basic-avatar) #:food-list (list (carrot #:amount-in-world 10) (fish #:amount-in-world 10)) #:crafter-list (list (my-cauldron)))
7.15 Weapon Crafter 1 Kata (difficulty = 2)
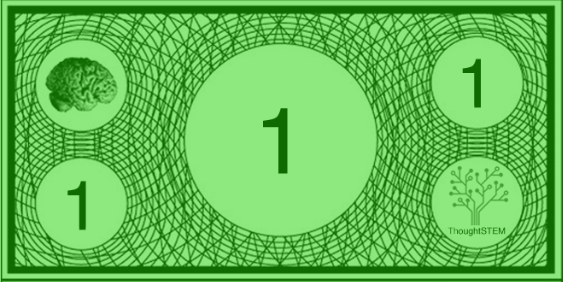
read
Code a game with a sword recipe that has a short build time, and a wooden table that produces swords.
code
#lang adventure (define my-sword-recipe (recipe #:product (sword) #:build-time 20)) (adventure-game #:avatar (basic-avatar) #:crafter-list (list (basic-crafter #:sprite woodtable-sprite #:recipe-list (list my-sword-recipe))))
7.16 Weapon Crafter 2 Kata (difficulty = 2)
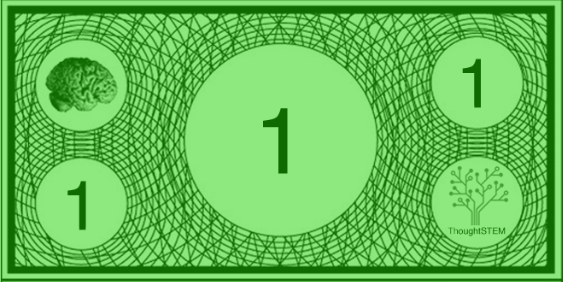
read
Code a game with a sword recipe that has a moderately long build time, and a wooden table that produces strong swords.
code
#lang adventure (define my-sword-recipe (recipe #:product (sword #:name "Heavy Sword" #:damage 100) #:build-time 40)) (adventure-game #:avatar (basic-avatar) #:crafter-list (list (basic-crafter #:sprite woodtable-sprite #:recipe-list (list my-sword-recipe))))
7.17 Weapon Crafter 3 Kata (difficulty = 3)
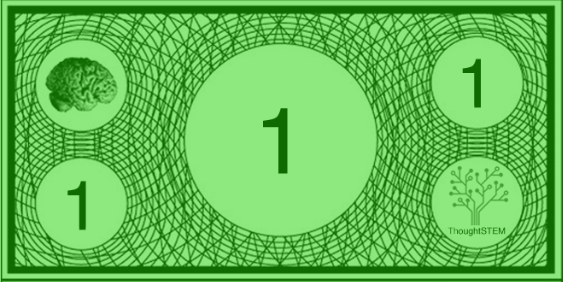
read
Code a game with 10 valuable coins, an expensive fire magic recipe that has a long build time, and a wooden table that produces fast fire magic.
code
#lang adventure (define my-fire-magic-recipe (recipe #:product (fire-magic #:name "Fire Magic" #:speed 5) #:build-time 60 #:cost 100)) (adventure-game #:avatar (basic-avatar) #:coin-list (list (basic-coin #:value 20 #:amount-in-world 10)) #:crafter-list (list (basic-crafter #:sprite woodtable-sprite #:recipe-list (list my-fire-magic-recipe))))
7.18 Sky 1 Kata (difficulty = 1)
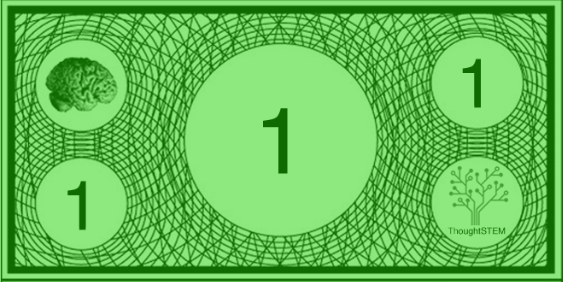
read
Code a game with a sky that has a long day.
code
#lang adventure (adventure-game #:avatar (basic-avatar) #:sky (basic-sky #:length-of-day 5000))
7.19 Sky 2 Kata (difficulty = 1)
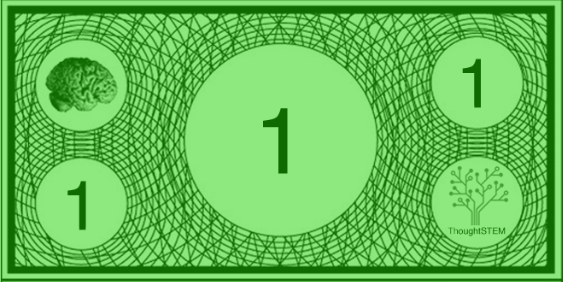
read
Code a game with a sky that has a short day and an extremely dark night.
code
#lang adventure (adventure-game #:avatar (basic-avatar) #:sky (basic-sky #:length-of-day 500 #:max-darkness 255))
7.20 Sky 3 Kata (difficulty = 1)
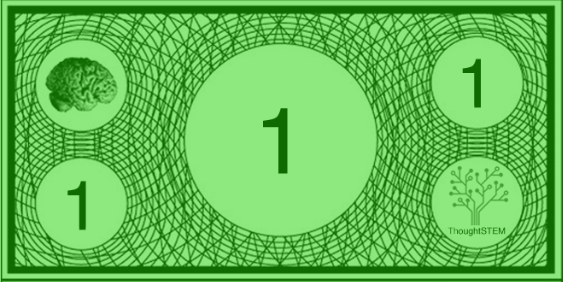
read
Code a game with a sky that has a light night with a magenta night sky.
code
#lang adventure (adventure-game #:avatar (basic-avatar) #:sky (basic-sky #:night-sky-color 'darkmagenta #:max-darkness 150))
7.21 Sky 4 Kata (difficulty = 1)
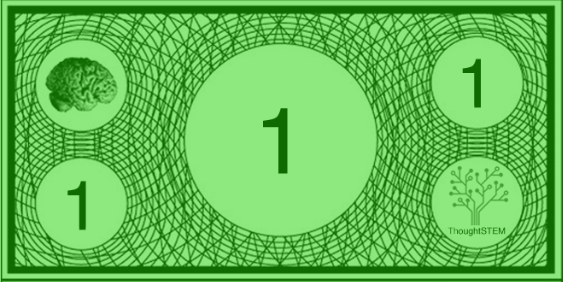
read
Code a game with 20 nocturnal enemies and a sky. Make the sky have a short night.
code
#lang adventure (adventure-game #:avatar (basic-avatar) #:enemy-list (list (basic-enemy #:amount-in-world 20 #:night-only? #t)) #:sky (basic-sky #:length-of-day 2400 #:start-of-daytime 200 #:end-of-daytime 2200))
7.22 Bg 1 Kata (difficulty = 1)
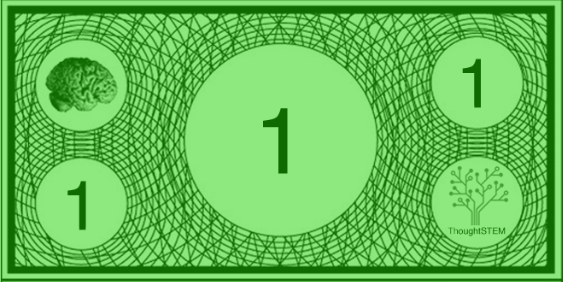
read
Code a game with a background.
code
#lang adventure (adventure-game #:avatar (basic-avatar) #:bg (basic-bg))
7.23 Bg 2 Kata (difficulty = 1)
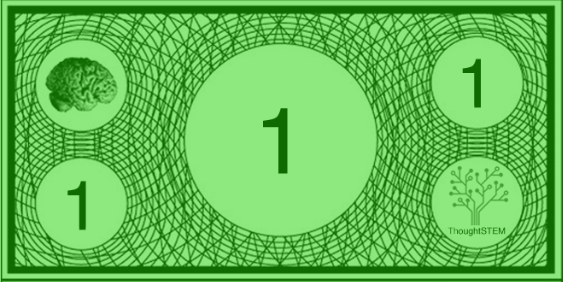
read
Code a game with a desert background.
code
#lang adventure (adventure-game #:avatar (basic-avatar) #:bg (basic-bg #:image DESERT-BG))
7.24 Bg 3 Kata (difficulty = 2)
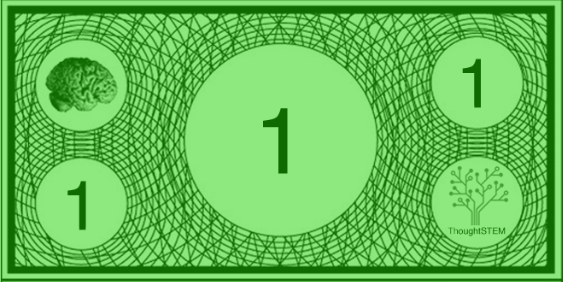
read
Code a game with a lava background that has 2 rows and 2 columns.
code
#lang adventure (define (my-bg) (basic-bg #:image LAVA-BG #:rows 2 #:columns 2)) (adventure-game #:avatar (basic-avatar) #:bg (my-bg))
7.25 Bg 4 Kata (difficulty = 1)
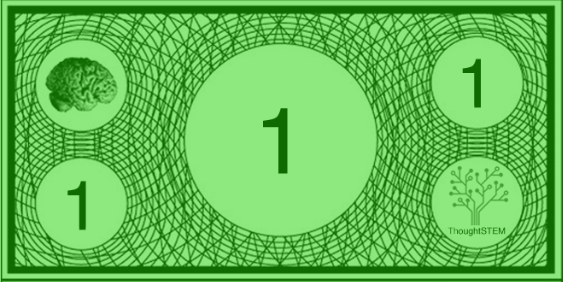
read
Code a game with a high-definition lava background that has 2 rows, 2 columns, and starts on tile 3.
code
#lang adventure (define (my-bg) (basic-bg #:image LAVA-BG #:rows 2 #:columns 2 #:start-tile 3 #:hd? #t)) (adventure-game #:bg (my-bg))