5 Food Katas
5.1 Food 1 Kata (difficulty = 1)
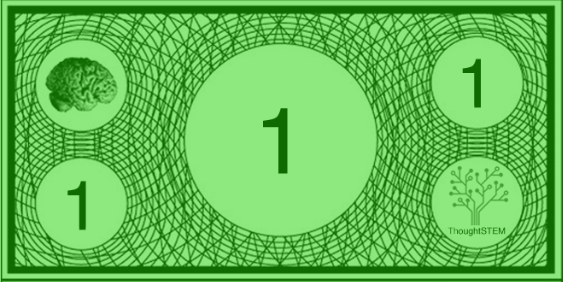
read
Code a game with several hearty foods.
code
#lang adventure (adventure-game #:food-list (list (basic-food #:sprite apples-sprite #:name "Apples" #:amount-in-world 10 #:heals-by 20)))
5.2 Food 2 Kata (difficulty = 2)
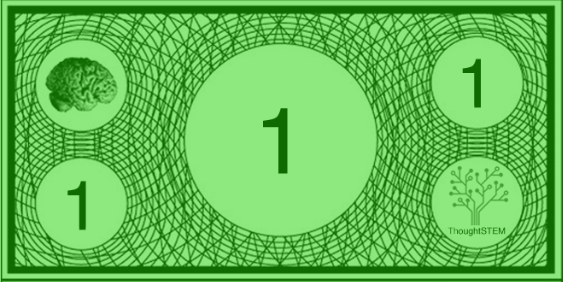
read
Code a game with 2 types of food: a common apple; and a very rare, hearty cherry.
code
#lang adventure (define my-food (basic-food #:sprite apples-sprite #:name "Apples" #:amount-in-world 10)) (define special-food (basic-food #:sprite cherry-sprite #:name "Cherry" #:heals-by 50 #:respawn? #f)) (adventure-game #:food-list (list my-food special-food))
5.3 Food 3 Kata (difficulty = 2)
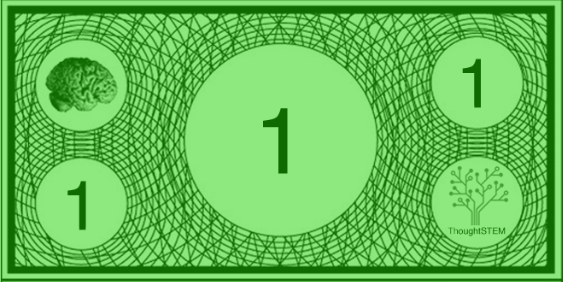
read
Code a game with an avatar who starts at half-health. Add a hearty steak that spawns a cutscene on pickup. The cutscene includes the steak sprite and text.
code
#lang adventure (define special-food (basic-food #:sprite steak-sprite #:name "Rare Steak" #:heals-by 100 #:on-pickup (spawn (page (set-sprite-scale 2 steak-sprite) "This tastes raw ..." "maybe I should have cooked it.")))) (adventure-game #:avatar (basic-avatar #:health 100 #:max-health 200) #:food-list (list special-food))
5.4 Food 4 Kata (difficulty = 3)
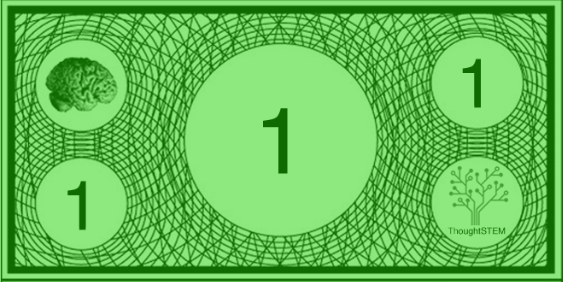
read
Code a game with several fish and a crafter that produces a hearty fish stew.
code
#lang adventure (define fish-stew (basic-food #:name "Fish Stew" #:sprite fishstew-sprite #:respawn? #f #:heals-by 50)) (define fish-stew-recipe (recipe #:product fish-stew #:build-time 40 #:ingredients (list "Fish"))) (adventure-game #:food-list (list (fish #:amount-in-world 10)) #:crafter-list (list (basic-crafter #:recipe-list (list fish-stew-recipe))))
5.5 Food 5 Kata (difficulty = 5)
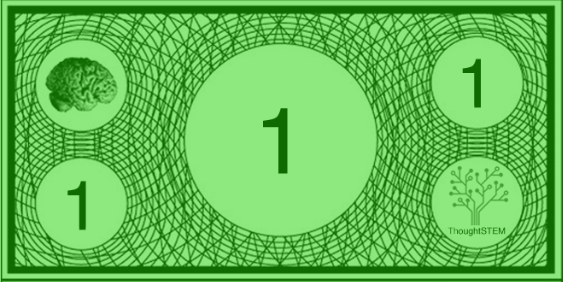
read
Code a game with an NPC and a crafter that produces a carrot stew. Give the NPC dialog and a quest to make the carrot stew.
code
#lang adventure (define carrot-stew (basic-food #:name "Carrot Stew" #:sprite carrotstew-sprite)) (define my-carrot-stew-recipe (recipe #:product carrot-stew #:ingredients (list "Carrot"))) (adventure-game #:npc-list (list (basic-npc #:dialog (list "Can you make me some carrot stew?") #:quest-list (list (craft-quest #:item carrot-stew)))) #:food-list (list (carrot)) #:crafter-list (list (basic-crafter #:recipe-list (list my-carrot-stew-recipe))))