4 Npc Katas
4.1 Npc 1 Kata (difficulty = 1)
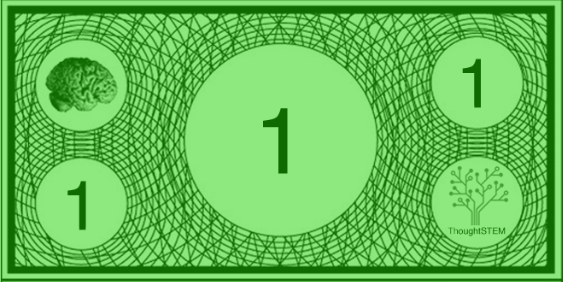
Tip: The NPC modes are ’still, ’follow, ’wander (the default), and ’pace.
read
Code a game with a witch NPC who follows you. Give her a name and a start location.
code
#lang adventure (adventure-game #:npc-list (list (basic-npc #:sprite witch-sprite #:name "Witch" #:mode 'follow #:position (posn 200 200) #:tile 3)))
4.2 Npc 2 Kata (difficulty = 4)
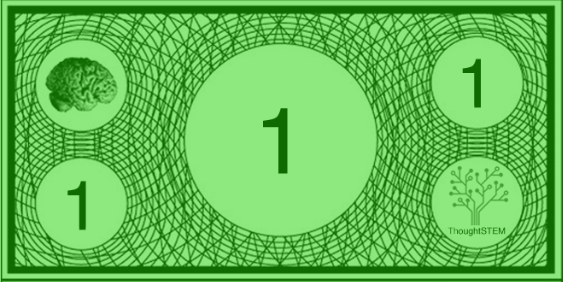
read
Code a game with 2 NPCs. Give both NPCs names and multiple lines of dialog.
code
#lang adventure (define (polite-npc) (basic-npc #:name "Riley" #:dialog (list "Oh hello there! I'm Riley." "Beautiful day today!"))) (define (rude-npc) (basic-npc #:name "..." #:dialog (list "Woah, who are you??" "Nevermind -- I'm too busy." "Move along, now!"))) (adventure-game #:npc-list (list (polite-npc) (rude-npc)))
4.3 Npc 3 Kata (difficulty = 4)
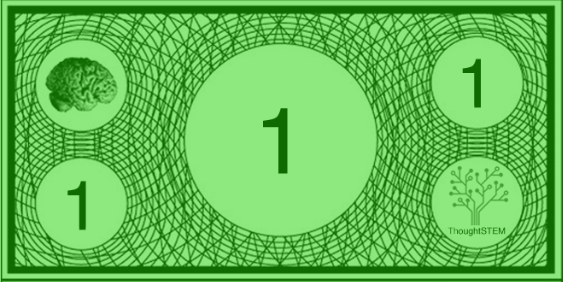
read
Code a game with an avatar and a named NPC. Give the avatar 3 questions and the NPC 3 responses.
code
#lang adventure (define player-dialog (player-dialog-with "Jordan" #:dialog-list (list "Hello. What's your name?" "Are you lost?" "What's the meaning of life?"))) (define npc-response (list (list "I'm Jordan," "but you can call me J.") (list "Nope. Just exploring!") (list "That's a strange question..."))) (adventure-game #:avatar (basic-avatar #:components player-dialog) #:npc-list (list (basic-npc #:name "Jordan" #:dialog npc-response)))
4.4 Npc 4 Kata (difficulty = 3)
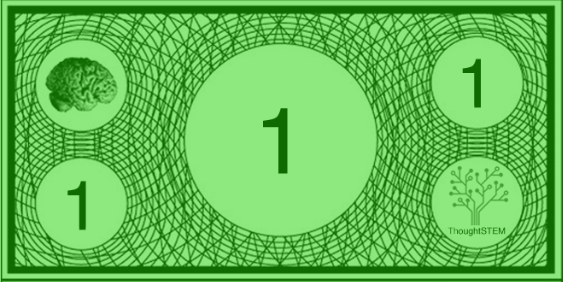
read
Code a game with an avatar who has random dialog and 2 NPCs with random responses.
code
#lang adventure (adventure-game #:avatar (basic-avatar #:components (random-player-dialog-with "Dakota") (random-player-dialog-with "Alex")) #:npc-list (list (basic-npc #:name "Dakota" #:dialog (random-npc-response)) (basic-npc #:name "Alex" #:dialog (random-npc-response))))
4.5 Npc 5 Kata (difficulty = 3)
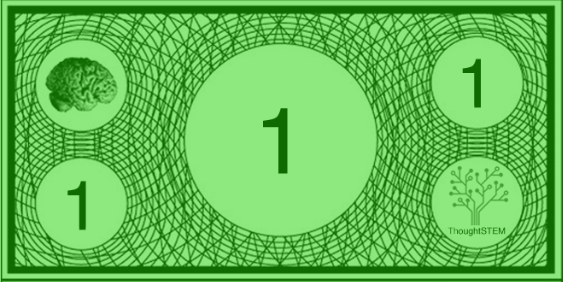
read
Code a game with an NPC who has a quest to fetch their lost spear. Give the NPC new dialog after the quest is complete.
code
#lang adventure (define spear-quest (fetch-quest #:item (spear) #:quest-complete-dialog (list "YAY! MY SPEAR!") #:new-response-dialog (list "Thanks again!"))) (adventure-game #:npc-list (list (basic-npc #:name "Charlie" #:dialog (list "Can you find my spear?") #:quest-list (list spear-quest))))