6 Fetch Quest Katas
6.1 Fetch Quest 1 Kata (difficulty = 2)
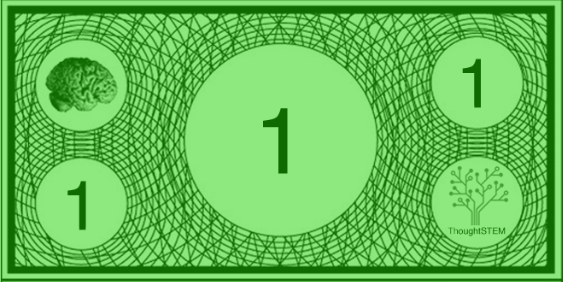
read
Code a game with an NPC who has a fetch quest to find their lost cat.
code
#lang adventure (define lost-cat (basic-item #:name "Mylo" #:sprite cat-sprite)) (adventure-game #:npc-list (list (basic-npc #:dialog (list "Can you help me find my cat?" "I've looked everywhere!") #:quest-list (list (fetch-quest #:item lost-cat)))))
6.2 Fetch Quest 2 Kata (difficulty = 3)
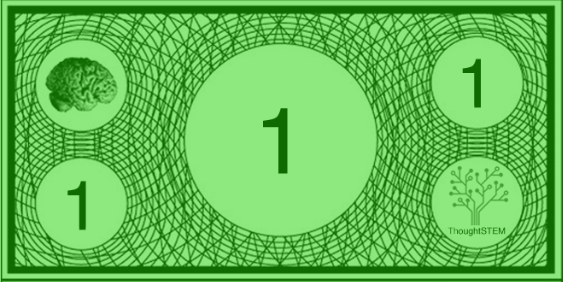
read
Code a game with an NPC who has a quest to find their lost sword. Give the quest an ending cutscene including the NPC sprite and some text.
code
#lang adventure (define my-cutscene (basic-cutscene (page (set-sprite-scale 2 darkknight-sprite) "Thank you! I should probably stop losing this..."))) (adventure-game #:npc-list (list (basic-npc #:sprite darkknight-sprite #:dialog (list "Can you help me find my sword?") #:quest-list (list (fetch-quest #:item (sword) #:cutscene my-cutscene)))))
6.3 Fetch Quest 3 Kata (difficulty = 3)
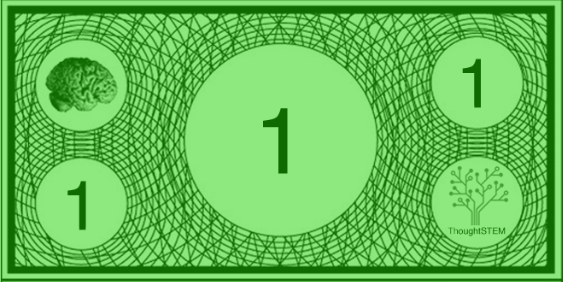
read
Code a game with an NPC who has 2 quests to return 2 different pets. Add a reward to both of the quests.
code
#lang adventure (define fetch-quest-1 (fetch-quest #:item (basic-item #:name "Mylo" #:sprite cat-sprite) #:reward-amount 200)) (define fetch-quest-2 (fetch-quest #:item (basic-item #:name "Buttons" #:sprite whitecat-sprite) #:reward-amount 200)) (adventure-game #:npc-list (list (basic-npc #:name "Erin" #:sprite lightelf-sprite #:dialog (list "Can you help me find my cats?" "I'll give you a reward for each cat.") #:quest-list (list fetch-quest-1 fetch-quest-2))))
6.4 Fetch Quest 4 Kata (difficulty = 3)
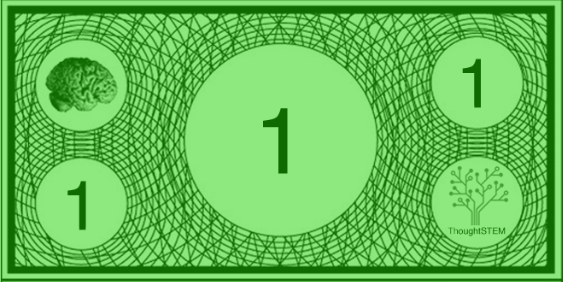
read
Code a game with an NPC who has a rewarded quest to find their antique bowl. The NPC’s dialog changes once the quest is complete.
code
#lang adventure (define my-fetch-quest (fetch-quest #:item (basic-item #:sprite bowl-sprite) #:quest-complete-dialog (list "Phew! This is a family herloom!!") #:new-response-dialog (list "Thanks again! My mom was so mad...") #:reward-amount 400)) (adventure-game #:npc-list (list (basic-npc #:name "Erin" #:sprite lightelf-sprite #:dialog (list "Can you find my antique bowl?") #:quest-list (list my-fetch-quest))))
6.5 Fetch Quest 5 Kata (difficulty = 4)
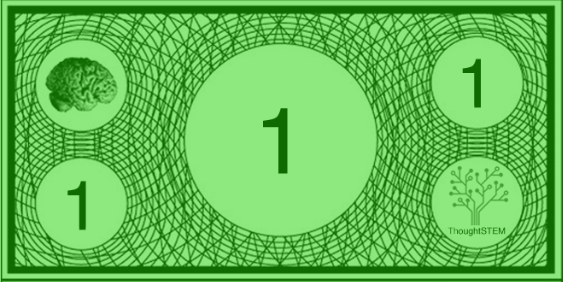
read
Code a game with an NPC who has a high-reward quest to find their lost cat. Give the cat pickup and drop cutscenes (the drop cutscene only happens if dropped away from the NPC).
code
#lang adventure (define my-quest-item (basic-item #:name "Mylo" #:sprite cat-sprite #:on-store (spawn (page (set-sprite-scale 2 cat-sprite) "This must be Erin's lost cat")) #:on-drop (spawn (page "Maybe I shouldn't leave him" "alone like this.") #:rule (not/r (entity-in-game? "Erin"))))) (adventure-game #:npc-list (list (basic-npc #:name "Erin" #:sprite lightelf-sprite #:dialog (list "Can you help my find my cat?") #:quest-list (fetch-quest #:item my-quest-item #:reward-amount 500))))